From what I understand, a web api controller will automatically
convert the json request and map the parameters, so is it best to
create a custom class
Yes, If the data passed with the request can be mapped to the object, Web API will convert the JSON or XML object to the class object.
If you want your json data to come inside an object to your method then first define a class which will hold your json data.
public class UserListRequest
{
public int UserId { get; set; }
public string CsvListOfIds { get; set; }
}
Later modify your method signature to receive object of that class :
[HttpPost]
public List<User> GetUserList(UserListRequest obj)
{
List<User> list = new List<User>();
list.Add(new User { ID = obj.UserId });
list.Add(new User { ID = obj.UserId + 1 }); //something
return list;
}
Remember to use [HttpPost]
attribute. Also there should be one post method you may comment out the already provided Post
method in the controller. (If you want to have multiple post methods inside your controller then see this question)
You can send the request through jquery or Fiddler (or any way you want). I tested it with Fiddler. You may see How to debug a Web API through Fiddler
Once your controller is setup, then build your project and start it in debug mode. Start Fiddler and go to composer.
There paste the URI of your controller (from IE/Brower) in address bar, and select POST
as Http method.
Your URI would be like:
http://localhost:60291/api/values/GetUserList
In Fiddler Composer -> Request Header specify
Content-Type: application/json
In Fiddler Composer -> Request Body, specify your JSON
{
"UserId": 1,
"CsvListOfIds": "2,3,4,5"
}
(You can also create your C# template class from json using http://json2csharp.com/)
Put a debug point on your method in the controller and then in Fiddler Hit Execute
, you will see that your Debug point in Visual studio is Hit and data is populated in the parameter.
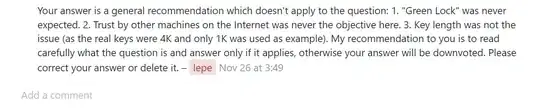
Later you can return the List<User>
, and you will see the response in Fiddler.
[{"ID":1},{"ID":2}]
Temporarily I created a class User
like:
public class User
{
public int ID { get; set; }
}
Hope this helps.