If you want to split a string based on the occurrence of a substring (such as "\r\n") you will have to use string search functions such as char * strstr(,). strstr() will return a pointer to the matched sub-string. You can then then manipulate the resulting string at that point to remove strlen("\r\n") bytes from the resultant, and continue your search.
[EDITED] from an excellent post here with a reference to excellent code by this guy
m-qayyums code is called in a loop to show you how you can look for (and replace) multiple occurrences of a sub-string with another sub-string, even an empty string using strstr(,) et. al.
#include <ansi_c.h>
#include <stdio.h>
#include <string.h>
char *replace_str(char *str, char *orig, char *rep);
int main ()
{
char origStr[]={"this is anggg originalggg lineggg ogggf texgggt"};
char newString[]={"this is anggg originalggg lineggg ogggf texgggt"};
sprintf(newString, "%s", origStr);
while(strstr(newString, "ggg"))
{
sprintf(newString, "%s", replace_str(newString, "ggg", ""));
}
printf("Original String: %s\n", origStr);
printf("New String: %s\n", newString);
getchar();
return 0;
}
char *replace_str(char *str, char *orig, char *rep)
{
static char buffer[4096];
char *p;
if(!(p = strstr(str, orig))) // Is 'orig' even in 'str'?
return str;
strncpy(buffer, str, p-str); // Copy characters from 'str' start to 'orig' st$
buffer[p-str] = '\0';
sprintf(buffer+(p-str), "%s%s", rep, p+strlen(orig));
return buffer;
}
[EDIT_2] replacing "ggg" with "\r\n" I got this result using the code above:
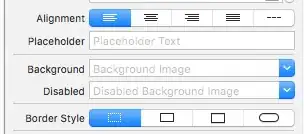