Yes, I'm really late :)
But I would like to share one more alternative solution. The idea is just to hide the grouped menu items when the SearchView
gets expanded.
Step 1:
Group your menu items. Please make sure showAsAction
of search view item is app:showAsAction="collapseActionView|always"
<group android:id="@+id/myMenuGroup">
<item
android:id="@+id/actionSearch"
android:icon="@drawable/ic_search"
android:title="@string/search"
app:actionViewClass="android.widget.SearchView"
app:iconTint="@color/textColorVariant2"
app:showAsAction="collapseActionView|always" />
<item
android:id="@+id/actionFilter"
android:icon="@drawable/ic_filter"
android:orderInCategory="0"
android:title="@string/filter"
app:iconTint="@color/textColorVariant2"
app:showAsAction="ifRoom" />
..... </group>
Step 2: Get the menu reference in onPrepareOptionsMenu()
callback
override fun onPrepareOptionsMenu(menu: Menu?): Boolean {
super.onPrepareOptionsMenu(menu)
this.menu = menu // this.menu is a global scoped variable
return true
}
Step 3
Add MenuItem.OnActionExpandListener
into your activity and implement onMenuItemActionExpand()
and onMenuItemActionExpand()
like below
override fun onMenuItemActionExpand(p0: MenuItem?): Boolean {
menu?.setGroupVisible(R.id.myMenuGroup, false)
return true
}
override fun onMenuItemActionCollapse(p0: MenuItem?): Boolean {
menu?.setGroupVisible(R.id.myMenuGroup, true)
return true
}
This approach will expand the SearchView
completely inside the ActionBar
even if there are other menu
icons present
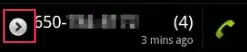
