As Luv said this is an old question but I've found two more solutions that may be helpful.
I'm using the sys.dm_sql_referenced_entities system object that finds all referenced objects and columns in a specified object. You can use the following query:
SELECT DISTINCT
referenced_schema_name AS SchemaName,
referenced_entity_name AS TableName,
referenced_minor_name AS ColumnName
FROM sys.dm_sql_referenced_entities ('yourrefencingobject', 'OBJECT');
GO
Which gives the following result:
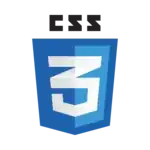
Downside of this object is that you'll need to specify a referencing object.
Or do a search like:
SELECT DISTINCT object_name(id)
FROM AdventureWorks2012.dbo.syscomments (nolock)
WHERE text like '%BusinessEntityID%'
Which gives the following result:
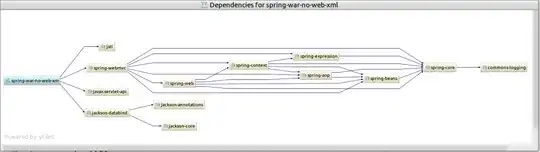
I've also find the following SP that you could use in this article, but haven't tested it properly:
> DECLARE @string varchar(1000), @ShowReferences char(1)
>
> SET @string = 'Person.Person.BusinessEntityID' --> searchstring
>
> SET @ShowReferences = 'N'
> /****************************************************************************/ /*
> */ /* TITLE: sp_FindReferences */ /* */ /* DATE: 18 February, 2004 */ /* */ /* AUTHOR: WILLIAM MCEVOY */ /* */ /****************************************************************************/ /*
> */ /* DESCRIPTION: SEARCH SYSCOMMENTS FOR INPUT STRING, OUTPUT NAME OF OBJECT */ /*
> */ /****************************************************************************/ set nocount on
>
> declare @errnum int ,
> @errors char(1) ,
> @rowcnt int ,
> @output varchar(255)
>
> select @errnum = 0 ,
> @errors = 'N' ,
> @rowcnt = 0 ,
> @output = ''
>
> /****************************************************************************/ /* INPUT DATA VALIDATION
> */ /****************************************************************************/
>
>
> /****************************************************************************/ /* M A I N P R O C E S S I N G
> */ /****************************************************************************/
>
> -- Create temp table to hold results DECLARE @Results table ( Name varchar(55), Type varchar(12), DateCreated datetime,
> ProcLine varchar(4000) )
>
>
> IF (@ShowReferences = 'N') BEGIN insert into @Results select
> distinct
> 'Name' = convert(varchar(55),SO.name),
> 'Type' = SO.type,
> crdate,
> ''
> from sysobjects SO
> join syscomments SC on SC.id = SO.id where SC.text like '%' + @string + '%' union select distinct
> 'Name' = convert(varchar(55),SO.name),
> 'Type' = SO.type,
> crdate,
> ''
> from sysobjects SO where SO.name like '%' + @string + '%' union select distinct
> 'Name' = convert(varchar(55),SO.name),
> 'Type' = SO.type,
> crdate,
> ''
> from sysobjects SO
> join syscolumns SC on SC.id = SO.ID where SC.name like '%' + @string + '%' order by 2,1 END ELSE BEGIN insert into @Results
> select
> 'Name' = convert(varchar(55),SO.name),
> 'Type' = SO.type,
> crdate,
> 'Proc Line' = text
> from sysobjects SO
> join syscomments SC on SC.id = SO.id where SC.text like '%' + @string + '%' union select
> 'Name' = convert(varchar(55),SO.name),
> 'Type' = SO.type,
> crdate,
> 'Proc Line' = ''
> from sysobjects SO where SO.name like '%' + @string + '%' union select
> 'Name' = convert(varchar(55),SO.name),
> 'Type' = SO.type,
> crdate,
> 'Proc Line' = ''
> from sysobjects SO
> join syscolumns SC on SC.id = SO.ID where SC.name like '%' + @string + '%' order by 2,1 END
>
> IF (@ShowReferences = 'N') BEGIN select Name,
> 'Type' = Case (Type)
> when 'P' then 'Procedure'
> when 'TR' then 'Trigger'
> when 'X' then 'Xtended Proc'
> when 'U' then 'Table'
> when 'C' then 'Check Constraint'
> when 'D' then 'Default'
> when 'F' then 'Foreign Key'
> when 'K' then 'Primary Key'
> when 'V' then 'View'
> else Type
> end,
> DateCreated
> from @Results
> order by 2,1 END ELSE BEGIN select Name,
> 'Type' = Case (Type)
> when 'P' then 'Procedure'
> when 'TR' then 'Trigger'
> when 'X' then 'Xtended Proc'
> when 'U' then 'Table'
> when 'C' then 'Check Constraint'
> when 'D' then 'Default'
> when 'F' then 'Foreign Key'
> when 'K' then 'Primary Key'
> when 'V' then 'View'
> else Type
> end,
> DateCreated,
> ProcLine
> from @Results
> order by 2,1 END
Hope this helps