Search by Image, keywords "Minecraft launcher", I would guess:
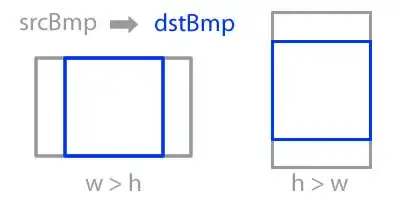
import java.awt.*;
import java.awt.image.*;
import java.io.IOException;
import javax.imageio.*;
import javax.swing.*;
class TexturePaintTest {
public JComponent makeUI() {
BufferedImage bi = null;
try {
bi = ImageIO.read(getClass().getResource("dirt_32x32.jpg"));
} catch(IOException ioe) {
ioe.printStackTrace();
throw new RuntimeException(ioe);
}
final TexturePaint texture = new TexturePaint(
bi, new Rectangle(bi.getWidth(),bi.getHeight()));
JPanel p = new JPanel(new BorderLayout()) {
@Override public void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D)g;
g2.setPaint(texture);
g2.fillRect(0, 0, getWidth(), getHeight());
g2.setPaint(new GradientPaint(
0, 0, new Color(0f,0f,0f,.2f),
0, getHeight(), new Color(0f,0f,0f,.8f), true));
g2.fillRect(0, 0, getWidth(), getHeight());
super.paintComponent(g);
}
};
p.setOpaque(false);
JPanel panel = new JPanel(new BorderLayout());
panel.setOpaque(false);
panel.add(makeLoginPanel(), BorderLayout.EAST);
p.add(panel, BorderLayout.SOUTH);
return p;
}
private JPanel makeLoginPanel() {
GridBagConstraints c = new GridBagConstraints();
JPanel p = new JPanel(new GridBagLayout());
p.setOpaque(false);
c.gridheight = 1;
c.gridwidth = 1;
c.gridy = 0;
c.gridx = 0;
c.weightx = 0.0;
c.insets = new Insets(2, 2, 2, 0);
c.anchor = GridBagConstraints.WEST;
p.add(makeLabel("Username:"), c);
c.gridx = 1;
c.weightx = 1.0;
c.fill = GridBagConstraints.HORIZONTAL;
p.add(new JTextField(12), c);
c.gridx = 2;
c.weightx = 0.0;
c.insets = new Insets(2, 2, 2, 2);
c.anchor = GridBagConstraints.WEST;
p.add(new JButton("Option"), c);
c.gridy = 1;
c.gridx = 0;
c.weightx = 0.0;
c.insets = new Insets(2, 2, 2, 0);
c.anchor = GridBagConstraints.WEST;
p.add(makeLabel("Password:"), c);
c.gridx = 1;
c.weightx = 1.0;
c.fill = GridBagConstraints.HORIZONTAL;
p.add(new JPasswordField(12), c);
c.gridx = 2;
c.weightx = 0.0;
c.insets = new Insets(2, 2, 2, 2);
c.anchor = GridBagConstraints.WEST;
p.add(new JButton("Login"), c);
return p;
}
private JLabel makeLabel(String str) {
JLabel l = new JLabel(str);
l.setForeground(Color.WHITE);
return l;
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override public void run() {
createAndShowGUI();
}
});
}
public static void createAndShowGUI() {
JFrame f = new JFrame();
f.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
f.getContentPane().add(new TexturePaintTest().makeUI());
f.setSize(320, 240);
f.setLocationRelativeTo(null);
f.setVisible(true);
}
}