You can do what you ask with a few intermediate steps. First convert your float to an int, then convert that int into a binary representation. From there, you can assign the resultant values to your bit field. This answer only addresses the intermediate steps.
The information here provides background and corroboration that 5.2 float
is represented by 01000000101001100110011001100110
. Decomposing a float into a binary representation can be done many different ways. This is only one implementation or representation. Reversing this process (i.e. going from binary back to float) would require following the same set of rules laid out in the link, backwards.
Note: endian is also a factor, I ran this in Windows/Intel environment.
Code:
#include <stdio.h> /* printf */
#include <stdlib.h> /* strtol */
const char *byte_to_binary32(long int x);
const char *byte_to_binary64(__int64 x);
int floatToInt(float a);
__int64 doubleToInt(double a);
int main(void)
{
long lVal, newInt;
__int64 longInt;
int i, len, array[65];
int len1, len2, len3, len4, len5, len6;
char buf[100];
char quit[]={" "};
float fNum= 5.2;
double dpNum= 5.2;
long double ldFloat;
while(quit[0] != 'q')
{
printf("\n\nEnter a float number: ");
scanf("%f", &fNum);
printf("Enter a double precision number: ");
scanf("%Lf", &ldFloat);
newInt = floatToInt(fNum);
{
//float
printf("\nfloat: %6.7f\n", fNum);
printf("int: %d\n", newInt);
printf("Binary: %s\n\n", byte_to_binary32(newInt));
}
longInt = doubleToInt(dpNum);
{
//double
printf("double: %6.16Lf\n", ldFloat);
printf("int: %lld\n", longInt);
printf("Binary: %s\n\n", byte_to_binary64(longInt));
/* byte to binary string */
sprintf(buf,"%s", byte_to_binary64(longInt));
}
len = strlen(buf);
for(i=0;i<len;i++)
{ //store binary digits into an array.
array[i] = (buf[i]-'0');
}
//Now you have an array of integers, either '1' or '0'
//you can use this to populate your bit field, but you will
//need more fields than you currently have.
printf("Enter any key to continue or 'q' to exit.");
scanf("%s", quit);
}
return 0;
}
const char *byte_to_binary32(long x)
{
static char b[33]; // bits plus '\0'
b[0] = '\0';
char *p = b;
unsigned __int64 z;
for (z = 2147483648; z > 0; z >>= 1) //2^32
{
*p++ = (x & z) ? '1' : '0';
}
return b;
}
const char *byte_to_binary64(__int64 x)
{
static char b[65]; // bits plus '\0'
b[0] = '\0';
char *p = b;
unsigned __int64 z;
for (z = 9223372036854775808; z > 0; z >>= 1) //2^64
{
*p++ = (x & z) ? '1' : '0';
}
return b;
}
int floatToInt(float a)
{
return (*((int*)&a));
}
__int64 doubleToInt(double a)
{
return (*((__int64*)&a));
}
Output
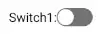