My example here is using the Boto3 library for Python 3 but I think it could still translate into this PHP question mainly because the core of the problem is not PHP specific but rather the HTML inside your HTML_BODY
field for SES and that is language agnostic so my example here should help.
This code successfully sends to my email address, formatted exactly how it appears in the example on W3 Schools here
HTML Example:
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
}
tr:nth-child(even){background-color: #f2f2f2}
</style>
</head>
<body>
<h2>Responsive Table</h2>
<p>A responsive table will display a horizontal scroll bar if the screen is too
small to display the full content. Resize the browser window to see the effect:</p>
<p>To create a responsive table, add a container element (like div) with <strong>overflow-x:auto</strong> around the table element:</p>
<div style="overflow-x:auto;">
<table>
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
</tr>
<tr>
<td>Jill</td>
<td>Smith</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
</tr>
<tr>
<td>Eve</td>
<td>Jackson</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
</tr>
<tr>
<td>Adam</td>
<td>Johnson</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
</tr>
</table>
</div>
</body>
</html>
AWS SES Example Using The Above HTML:
#Your Imports go here
import boto3
# Replace sender@example.com with your "From" address.
# This address must be verified with Amazon SES.
SENDER = "the_email_address_of_who_is_sending_this_email_out@gmail.com"
# Replace recipient@example.com with a "To" address. If your account
# is still in the sandbox, this address must be verified.
RECIPIENT = "the_email_address_of_who_will_be_receiving_this_email@gmail.com"
# Specify a configuration set. If you do not want to use a configuration
# set, comment the following variable, and the
# ConfigurationSetName=CONFIGURATION_SET argument below.
# CONFIGURATION_SET = "ConfigSet"
# If necessary, replace us-west-2 with the AWS Region you're using for Amazon SES.
AWS_REGION = "us-east-1"
# The subject line for the email.
SUBJECT = "ARL Data Lake"
# The email body for recipients with non-HTML email clients.
# BODY_TEXT = ("Amazon SES Test (Python)\r\n"
# "This email was sent with Amazon SES using the "
# "AWS SDK for Python (Boto)."
# )
BODY_TEXT = str(records)
# The HTML body of the email.
BODY_HTML = """<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
}
tr:nth-child(even){background-color: #f2f2f2}
</style>
</head>
<body>
<h2>Responsive Table</h2>
<p>A responsive table will display a horizontal scroll bar if the screen is too
small to display the full content. Resize the browser window to see the effect:</p>
<p>To create a responsive table, add a container element (like div) with <strong>overflow-x:auto</strong> around the table element:</p>
<div style="overflow-x:auto;">
<table>
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
<th>Points</th>
</tr>
<tr>
<td>Jill</td>
<td>Smith</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
<td>50</td>
</tr>
<tr>
<td>Eve</td>
<td>Jackson</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
<td>94</td>
</tr>
<tr>
<td>Adam</td>
<td>Johnson</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
<td>67</td>
</tr>
</table>
</div>
</body>
</html>"""
# The character encoding for the email.
CHARSET = "UTF-8"
# Create a new SES resource and specify a region.
client = boto3.client('ses', region_name=AWS_REGION)
# Try to send the email.
try:
# Provide the contents of the email.
response = client.send_email(
Destination={
'ToAddresses': [
RECIPIENT,
],
},
Message={
'Body': {
'Html': {
'Charset': CHARSET,
'Data': BODY_HTML,
},
'Text': {
'Charset': CHARSET,
'Data': BODY_TEXT,
},
},
'Subject': {
'Charset': CHARSET,
'Data': SUBJECT,
},
},
Source=SENDER,
# If you are not using a configuration set, comment or delete the
# following line
# ConfigurationSetName=CONFIGURATION_SET,
)
# Display an error if something goes wrong.
except Exception as e:
print(e.response['Error']['Message'])
else:
print("Email sent! Message ID:"),
print(response['MessageId'])
The Email Result:

Sources & Further Help/Examples:
Note:
To run this example easily I recommend setting up a quick Cloud9 IDE in AWS, installing Python 3 on it, copy and paste my code into it, then click run. If your Cloud9 has the proper roles and permissions to use SES then it should work. Also note that Cloud9 is just running on top of an EC2-Instance so you can SSH into it (directly from the Management Console on your browser which is nice) and run something like "sudo yum install python-3.6" and manually run the code using "sudo touch code_example.py", "sudo chmod 755 code_example.py", "sudo vi code_example.py", then hit the "i" key which stands for insert, then right click and for my SSH client that pastes the code I had copied onto my clipboard into the SSH's text editor for VI, then click "ESC" to get out of insert mode, then click ":wq" and click enter which stands for "write" and then "quit", then to run it do "python3 code_example.py". If you successfully installed Python it should work. But like I said it's easier to just use Cloud9, open the IDE for Cloud9, create a new Python file, paste the code in, then run it as Python 3 there's a button that does it just like any normal IDE.
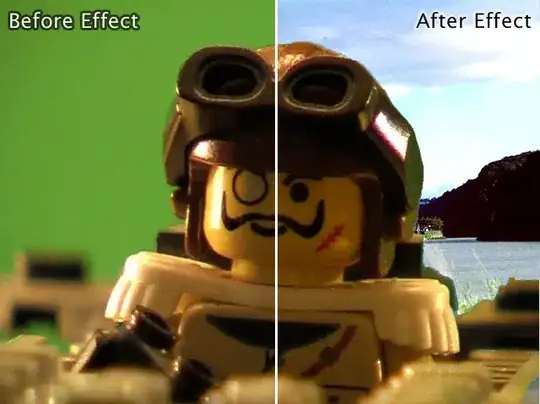