I created my own custom view to look like iOS UIAlertView 7. With that technique you can create a custom alert for both iOS 6 and iOS 7.
For that, I created a UIView in my xib file of my UIViewController :
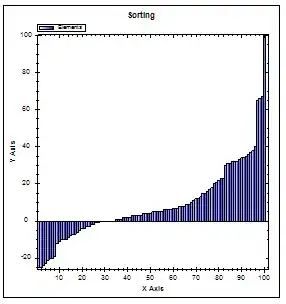
I added some @property for this view :
// Custom iOS 7 Alert View
@property (nonatomic, weak) IBOutlet UIView *supportViewPopup; // My UIView
@property (nonatomic, weak) IBOutlet UIView *supportViewPopupBackground; // The grey view
@property (nonatomic, weak) IBOutlet UIView *supportViewPopupAction; // The white view with outlets
// Property for customize the UI of this alert (you can add other labels, buttons, tableview, etc.
@property (nonatomic, weak) IBOutlet UIButton *buttonOK;
@property (nonatomic, weak) IBOutlet UIButton *buttonCancel;
@property (nonatomic, weak) IBOutlet UILabel *labelDescription;
On my viewDidLoad :
- (void)viewDidLoad
{
[super viewDidLoad];
// Support View
self.supportViewPopupAction.layer.cornerRadius = 5.0f;
self.supportViewPopupAction.layer.masksToBounds = YES;
// Add Support View
[self.view addSubview:self.supportViewPopup];
// Center Support view
self.supportViewPopup.center = self.view.center;
// Alpha
self.supportViewPopup.alpha = 0.0f;
self.supportViewPopupBackground.alpha = 0.0f;
self.supportViewPopupAction.alpha = 0.0f;
}
Action to display Popup :
- (IBAction)displayPopup
{
// Support View
self.supportViewPopup.alpha = 1.0f;
self.supportViewPopupBackground.alpha = 0.5f;
// Animation
[UIView animateWithDuration:0.5f
animations:^{
self.supportViewPopupAction.alpha = 1.0f;
}];
}
Action to dismiss Popup :
- (IBAction)dismissModal
{
// Animation
[UIView animateWithDuration:0.5f
animations:^{
self.supportViewPopup.alpha = 0.0f;
self.supportViewPopupBackground.alpha = 0.0f;
self.supportViewPopupAction.alpha = 0.0f;
}];
}
So, with that you can configure your supportViewPopupAction
like you want with buttons, table view, labels, collection view, etc...
I spent time to write this example of alert view. I hope this will help you !