Like I mentioned in my comment, if you know what all the separators are then it is a piece of cake.
For testing demonstration purpose I have this simple example.
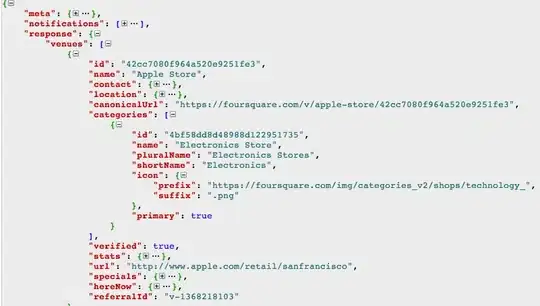
In this example, I will output the cell addresses of the matches in Column D
Since this is an example/demonstration, I am using Hardcoded values to loop through the column. To actually find the last row in a column, see THIS LINK
Logic:
- Store the separators
_
,.
,-
in a string
- Split it using "," as a delimiter and store it in an array
- When looping though the column values, simply loop through the array and replace the separators by ""
- Once you get the base string, simply use
.Find
to search Col A for that string.
Code:
Option Explicit
'~~> This is the list for your separators separated by comman
Const sep As String = "_,.,-"
Sub Sample()
Dim ws As Worksheet
Dim MyAr
Dim SearchString As String
Dim aCell As Range
Dim i As Long, j As Long
'~~> Split the separator using "," and store it in an array
MyAr = Split(sep, ",")
'~~> Set this to the relevant workbook
Set ws = ThisWorkbook.Sheets("Sheet1")
With ws
'~~> Loop through Col C
For i = 1 To 3
If Len(Trim(.Range("C" & i).Value)) <> 0 Then
SearchString = .Range("C" & i).Value
'~~> Loop through the array and replace all separator by ""
For j = LBound(MyAr) To UBound(MyAr)
SearchString = Replace(SearchString, MyAr(j), "")
Next
'~~> Find the result txt in Col B
Set aCell = .Columns(2).Find(What:=Trim(SearchString), LookIn:=xlValues, _
LookAt:=xlWhole, SearchOrder:=xlByRows, SearchDirection:=xlNext, _
MatchCase:=False, SearchFormat:=False)
'~~> If found then output to cell D
If Not aCell Is Nothing Then
.Range("D" & i).Value = "Found in Cell " & aCell.Address
End If
End If
Next i
End With
End Sub
Screenshot:
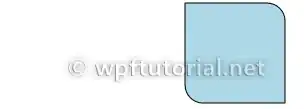