You can use unDelphiX to get a Delphi package that does DirectX.
You can download the package here: http://www.micrel.cz/Dx/
It is fully up to date and makes DirectX work almost like the standard GDI.
Drawing without flicker
The trick to eliminating flicker is to draw to an off-screen surface and "flip" the offscreen surface in view when you're done.
The flip can be synchronized with the VerticalSync, so there's no tearing as well.
Demo
There's a demo that displays the current time in milliseconds without any blinking.
unit Main;
interface
uses
Windows, Messages, SysUtils, Classes, Graphics, Controls, Forms, Dialogs,
StdCtrls, ExtCtrls, Menus, DXClass, DXDraws;
type
TMainForm = class(TDXForm)
DXDraw: TDXDraw;
DXTimer: TDXTimer; //Precision timer.
procedure DXDrawFinalize(Sender: TObject);
procedure DXDrawInitialize(Sender: TObject);
procedure DXTimerTimer(Sender: TObject; LagCount: Integer);
end;
var
MainForm: TMainForm;
implementation
uses MMSystem;
{$R *.DFM}
procedure TMainForm.DXDrawInitialize(Sender: TObject);
begin
DXTimer.Enabled := True;
end;
procedure TMainForm.DXDrawFinalize(Sender: TObject);
begin
DXTimer.Enabled := False;
end;
procedure TMainForm.DXTimerTimer(Sender: TObject; LagCount: Integer);
var
formattedDateTime: string;
begin
if not DXDraw.CanDraw then exit;
DXDraw.Surface.Fill(0);
with DXDraw.Surface.Canvas do //Draw to offscreen surface.
begin
Brush.Style := bsClear;
Font.Color := clWhite;
Font.Size := 30;
DateTimeToString(formattedDateTime, 'hh:nn:ss.zzz', Now);
Textout(30, 30, formattedDateTime);
Release; { Indispensability }
end;
DXDraw.Flip; //Flip the offscreen surface to screen, no flicker :=)
end;
end.
Installing unDelphiX in XE2
In order to get the package installed in Delphi XE2 I had to add DesignIDE
to the requires
clause of the dpk. like so:

This will fix the DesignIntf.dcu
not found error.
Displaying full screen
If you want to do fullscreen you enable it in the settings of DXDraw, but note:
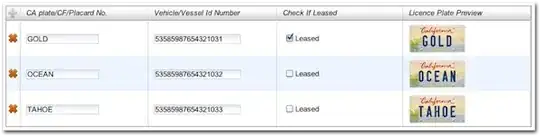
If you enable doFullScreen
without enabling doSelectDriver
you'll get an interface not supported
error. Enable them both and everything will be fine.
See: http://www.micrel.cz/Dx/history.rtf
Don't forget to listen for keypresses and exit the app on ESC or something, or you'll be stuck in full screen mode.