tl;dr
Duration.between( // Calculate time elapsed between two moments.
LocalDateTime // Represent a date with time-of-day but lacking the context of a time zone or offset-from-UTC.
.parse( "2013-10-17 15:45:01".replace( " " , "T" ) )
.atOffset( ZoneOffset.UTC ) // Returns an `OffsetDateTime` object.
.toInstant() , // Returns an `Instant` object.
Instant.now() // Capture the current moment as seen in UTC.
)
.toMinutes()
> 5
java.time
The other Answers are outdated, using terrible classes that were years ago supplanted by the modern java.time classes defined in JSR 310.
Parse your incoming string.
String input = "2013-10-17 15:45:01" ;
Modify the input to comply with ISO 8601. I suggest you educate the publisher of your data about the ISO 8601 standard.
String inoutModified = input.replace( " " , "T" ) ;
Parse as a LocalDateTime
because this input lacks an indicator of the intended offset or time zone.
LocalDateTime ldt = LocalDateTime.parse( input ) ;
I assume that input was intended to represent a moment as seen in UTC, with an offset of zero hours minutes seconds. If so, educate the publisher of your data about appending a Z
on the end to so indicate, per ISO 8601.
OffsetDateTime odt = ldt.atOffset( ZoneOffset.UTC ) ;
Extract an object of the simpler class, Instant
. This class is always in UTC.
Instant then = odt.toInstant() ;
Get current moment as seen in UTC.
Instant now = Instant.now() ;
Calculate the difference.
Duration d = Duration.between( then , now ) ;
Get duration as total whole minutes.
long minutes = d.toMinutes() ;
Test.
if ( minutes > 5 ) { … }
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?
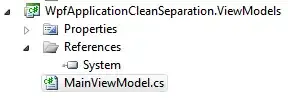