The code below demonstrates rotation of a float array by multiples of 90 degrees. I later converted the float array to a byte array so that I could generate test images to verify the results; I didn't include that part of the code because it only clutters the main point, and wasn't really what you were asking about. If you need that part, let me know I will post it.
Note that I did the rotation "out-of-place" rather than "in-place." I believe the latter is what you are really interested in, but even so, the approach below should be a good start for you. Additional discussion on the in-place transformation can be found here, which centers around the use of a transpose-type operation (memory swap), but I didn't have time to sort through all of those details, I leave that part for you.
Recall that for a counter-clockwise rotation of n*90 degrees, the transformation is given by:
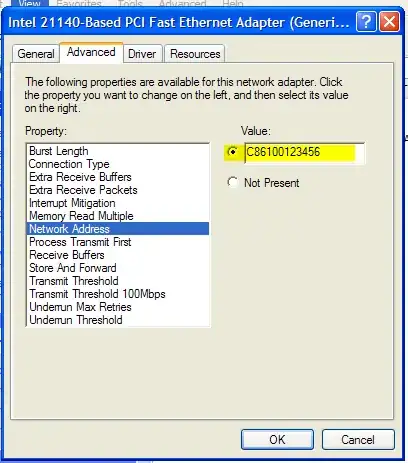
So then of course:

But in the actual code you will need to translate row' and col' by imageHeight/2 and imageWidth/2, respectively, as shown in the code below, to avoid passing negative indices to your arrays.
Denoting row_p and col_p as row' and col', the code looks like this:
// Note: nX = pixels wide, nY = pixels tall
float *dataVector = // some data source, arbitrary
// Setup the array for the out-of-place transformation:
float *dataVector2 = new float[nX*nY];
int n = -2; // Example: set n = -2 to rotate counter-clockwise 180 deg
for (int row = 0; row < nY; row++) {
for (int col = 0; col < nX; col++) {
int row_p = cosf(n*M_PI_2)*(row-nY/2) - sinf(n*M_PI_2)*(col-nX/2) + nY/2;
int col_p = sinf(n*M_PI_2)*(row-nY/2) + cosf(n*M_PI_2)*(col-nX/2) + nX/2;
dataVector2[row*nX + col] = dataVector[row_p*nX + col_p];
}
}
// Later convert float array to image ...
Note that in the code above I am using the rotated coordinates to access elements of the original array, and then mapping these values back to the original row, col coordinates:
dataVector2[row*nX + col] = dataVector[row_p*nX + col_p];
The result of this is that +n values give a clockwise rotation; if you want counter-clockwise rotations, simply pass in negative value of n, i.e., -n, to your code, as shown in the example above. The effect of this is to just change signs for the off-diagonal terms of the rotation matrix above.
Hope this helps.