As @mKorbel stated you can use HTML tags in Swing: How to Use HTML in Swing Components
Also you'll need a custom cell renderer: Using Custom Renderers
Example
This is just an example of implementation (it's not exactly what you need) but you can manage to make it more accurate:
table.setDefaultRenderer(Object.class, new DefaultTableCellRenderer(){
@Override
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
super.getTableCellRendererComponent(table, value, isSelected, hasFocus, row, column);
String str = value.toString();
String regex = ".*?user=\".*?\".*?";
Matcher matcher = Pattern.compile(regex).matcher(str);
if(matcher.matches()){
regex = "user=\".*?\"";
matcher = Pattern.compile(regex).matcher(str);
while(matcher.find()){
String aux = matcher.group();
str = str.replace(aux, "<b>" + aux + "</b>");
}
str = "<html>" + str + "</html>";
setText(str);
}
return this;
}
});
This renderer looks for user="whateverHere"
pattern in the string. If it matches then replaces all instances of this sub-string with the same sub-string sorrounded by <b></b>
tags. Finally sorrounds the whole text with <html></html>
tags.
More info about regex in this Q&A: Using Java to find substring of a bigger string using Regular Expression
As DefaultTableCellRenderer
extends from JLabel
(yes, a Swing component!) HTML tags will do the trick.
Screenshot
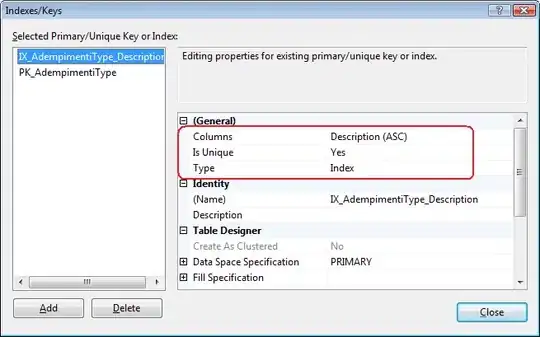