Howabout getLocation
, which returns the coordinate of a component on its parent component, or getLocationOnScreen
, which returns the coordinate of the component on the display?
Your second question about how x and y are calculated, I'm not sure what you mean by 'calculated'. The coordinates will be relative to something. Usually either the parent component (like a JPanel
on which the JButton
is sitting) or a location on the screen (such as getLocation
would return for a JFrame
).
A method like Point.distance
will subtract the two coordinates' x and y values and tell you the difference. This is just basic geometry.
For example, here is a method that will return the distance of a point from the center of a JButton
:
public static double getDistance(Point point, JComponent comp) {
Point loc = comp.getLocation();
loc.x += comp.getWidth() / 2;
loc.y += comp.getHeight() / 2;
double xdif = Math.abs(loc.x - point.x);
double ydif = Math.abs(loc.y - point.y);
return Math.sqrt((xdif * xdif) + (ydif * ydif));
}
This returns the hypotenuse of a triangle as a measurement in pixels, which means if the point you give it (like cursor coordinates) is at a diagonal it will give you the useful distance.
Point.distance
will do something like this.
I've noticed this old answer of mine has gotten quite a few views, so here is a better way to do the above (but doesn't really show the math):
public static double distance(Point p, JComponent comp) {
Point2D.Float center =
// note: use (0, 0) instead of (getX(), getY())
// if the Point 'p' is in the coordinates of 'comp'
// instead of the parent of 'comp'
new Point2D.Float(comp.getX(), comp.getY());
center.x += comp.getWidth() / 2f;
center.y += comp.getHeight() / 2f;
return center.distance(p);
}
Here's a simple example showing this kind of geometry in a Swing program:
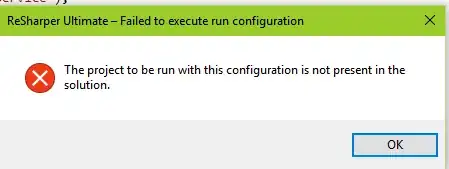
This draws a line to wherever the mouse cursor is and displays the length of the line (which is the distance from the center of the JPanel
to the cursor).
import javax.swing.*;
import java.awt.*;
import java.awt.geom.*;
import java.awt.event.*;
class DistanceExample implements Runnable {
public static void main(String[] args) {
SwingUtilities.invokeLater(new DistanceExample());
}
@Override
public void run() {
JLabel distanceLabel = new JLabel("--");
MousePanel clickPanel = new MousePanel();
Listener listener =
new Listener(distanceLabel, clickPanel);
clickPanel.addMouseListener(listener);
clickPanel.addMouseMotionListener(listener);
JPanel content = new JPanel(new BorderLayout());
content.setBackground(Color.white);
content.add(distanceLabel, BorderLayout.NORTH);
content.add(clickPanel, BorderLayout.CENTER);
JFrame frame = new JFrame();
frame.setContentPane(content);
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
static class MousePanel extends JPanel {
Point2D.Float mousePos;
MousePanel() {
setOpaque(false);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (mousePos != null) {
g.setColor(Color.red);
Point2D.Float center = centerOf(this);
g.drawLine(Math.round(center.x),
Math.round(center.y),
Math.round(mousePos.x),
Math.round(mousePos.y));
}
}
@Override
public Dimension getPreferredSize() {
return new Dimension(100, 100);
}
}
static class Listener extends MouseAdapter {
JLabel distanceLabel;
MousePanel mousePanel;
Listener(JLabel distanceLabel, MousePanel mousePanel) {
this.distanceLabel = distanceLabel;
this.mousePanel = mousePanel;
}
@Override
public void mouseMoved(MouseEvent e) {
Point2D.Float mousePos =
new Point2D.Float(e.getX(), e.getY());
mousePanel.mousePos = mousePos;
mousePanel.repaint();
double dist = distance(mousePos, mousePanel);
distanceLabel.setText(String.format("%.2f", dist));
}
@Override
public void mouseExited(MouseEvent e) {
mousePanel.mousePos = null;
mousePanel.repaint();
distanceLabel.setText("--");
}
}
static Point2D.Float centerOf(JComponent comp) {
Point2D.Float center =
new Point2D.Float((comp.getWidth() / 2f),
(comp.getHeight() / 2f));
return center;
}
static double distance(Point2D p, JComponent comp) {
return centerOf(comp).distance(p);
}
}