Found a method to do this by combining the answers here and here
The final solution is really counterintuitive to me.
It seems that you need to explicitly have a separate table name for ApplicationUser.
Also, you must make sure that base.OnModelCreating(modelBuilder) is called first
If you don't do it this way, the field will never honor the NOT NULL aspect of the [Required] attribute.
Here is the code I used which finally got this working:
public class ApplicationUser : IdentityUser
{
public string FirstName { get; set; }
}
public class ApplicationDbContext : IdentityDbContext<ApplicationUser>
{
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
//modelBuilder.Entity<IdentityUser>().ToTable("Users"); // Won't work if the table name is the same.
modelBuilder.Entity<ApplicationUser>().ToTable("Users")
.Property(p => p.FirstName).IsRequired();
}
// etc.
}
Which results in the following DB structure:
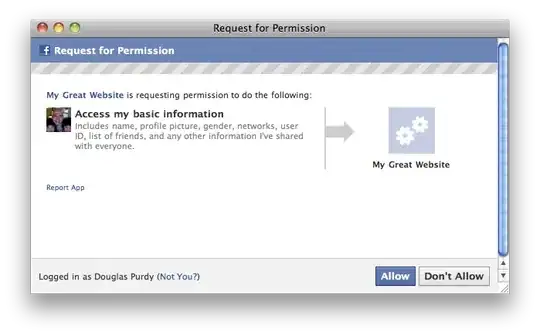