You can use stat_summary()
to calculate position of y
values as sum of depth
and use geom="text"
to add labels. The sum is used because your bars shows the sum of depth
values for each cut
value.
As suggest by @joran it is better to use stat_summary()
instead of geom_bar()
to show sums of y values because stat="identity"
makes problems due to overplotting of bars and if there will be negative values then bar will start in negative part of plot and end in positive part - result will be not the actual sum of values.
ggplot(diamonds[1:100,], aes(x = cut, y = depth)) +
facet_wrap(~ color) +
stat_summary(fun.y = sum, geom="bar", fill = "blue", aes(label=cut, vjust = 0)) +
stat_summary(fun.y = sum, geom="text", aes(label=cut), vjust = 0)
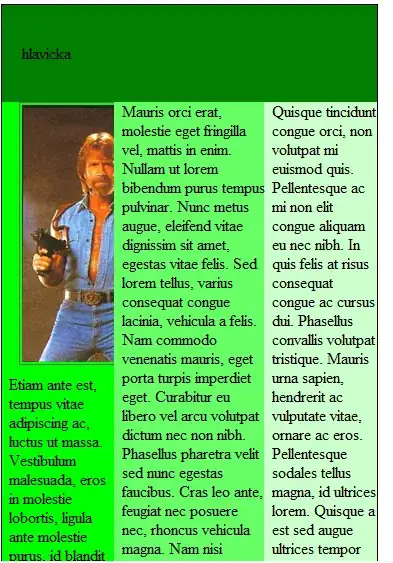
You can also precalculate sum of depth values and the you can use geom_bar()
with stat="identity"
and geom_text()
.
library(plyr)
diamonds2<-ddply(diamonds,.(cut,color),summarise,depth=sum(depth))
ggplot(diamonds2,aes(x=cut,y=depth))+
geom_bar(stat="identity",fill="blue")+
geom_text(aes(label=cut),vjust=0,angle=45,hjust=0)+
facet_wrap(~color)