The easiest solution is to use UseTouchAnimationsForAllNavigation
so that items manipulation in the view model does not cause the animation to occur at all.
<FlipView UseTouchAnimationsForAllNavigation="False" />
If the animation is important to you, then you can simply bind the value for UseTouchAnimationsForAllNavigation
in your view model, like this:
<FlipView UseTouchAnimationsForAllNavigation="{Binding ShowAnimations}" />
Does this make sense? If you do it in your view model (option 2) you can simply disable it while manipulating the collection, and then re-enable it so you get animations.
Here's a sample.
Using this code:
public class MyColorModel : BindableBase
{
Color _Color = default(Color);
public Color Color { get { return _Color; } set { SetProperty(ref _Color, value); } }
Visibility _Selected = Visibility.Collapsed;
public Visibility Selected { get { return _Selected; } set { SetProperty(ref _Selected, value); } }
public event EventHandler RemoveRequested;
public void RemoveMe()
{
if (RemoveRequested != null)
RemoveRequested(this, EventArgs.Empty);
}
public event EventHandler SelectRequested;
public void SelectMe()
{
if (SelectRequested != null)
SelectRequested(this, EventArgs.Empty);
}
}
public class MyViewModel : BindableBase
{
public MyViewModel()
{
this.Selected = this.Colors[1];
foreach (var item in this.Colors)
{
item.RemoveRequested += (s, e) =>
{
this.ShowAnimations = false;
this.Colors.Remove(s as MyColorModel);
this.ShowAnimations = true;
};
item.SelectRequested += (s, e) => this.Selected = s as MyColorModel;
}
}
ObservableCollection<MyColorModel> _Colors = new ObservableCollection<MyColorModel>(new[]
{
new MyColorModel{ Color=Windows.UI.Colors.Red },
new MyColorModel{ Color=Windows.UI.Colors.Green },
new MyColorModel{ Color=Windows.UI.Colors.Yellow },
new MyColorModel{ Color=Windows.UI.Colors.Blue },
new MyColorModel{ Color=Windows.UI.Colors.White },
new MyColorModel{ Color=Windows.UI.Colors.Brown },
new MyColorModel{ Color=Windows.UI.Colors.SteelBlue },
new MyColorModel{ Color=Windows.UI.Colors.Goldenrod },
});
public ObservableCollection<MyColorModel> Colors { get { return _Colors; } }
MyColorModel _Selected = default(MyColorModel);
public MyColorModel Selected
{
get { return _Selected; }
set
{
if (_Selected != null)
_Selected.Selected = Visibility.Collapsed;
value.Selected = Visibility.Visible;
SetProperty(ref _Selected, value);
}
}
bool _ShowAnimations = true;
public bool ShowAnimations { get { return _ShowAnimations; } set { SetProperty(ref _ShowAnimations, value); } }
}
public abstract class BindableBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected void SetProperty<T>(ref T storage, T value, [System.Runtime.CompilerServices.CallerMemberName] String propertyName = null)
{
if (!object.Equals(storage, value))
{
storage = value;
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
protected void RaisePropertyChanged([System.Runtime.CompilerServices.CallerMemberName] String propertyName = null)
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
Try this XAML:
<Page.DataContext>
<local:MyViewModel/>
</Page.DataContext>
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid.RowDefinitions>
<RowDefinition/>
<RowDefinition Height="150" />
</Grid.RowDefinitions>
<FlipView ItemsSource="{Binding Colors}" SelectedItem="{Binding Selected, Mode=TwoWay}" UseTouchAnimationsForAllNavigation="{Binding ShowAnimations}">
<FlipView.ItemTemplate>
<DataTemplate>
<Rectangle>
<Rectangle.Fill>
<SolidColorBrush Color="{Binding Color}" />
</Rectangle.Fill>
</Rectangle>
</DataTemplate>
</FlipView.ItemTemplate>
</FlipView>
<ItemsControl ItemsSource="{Binding Colors}" Grid.Row="1" VerticalAlignment="Top" HorizontalAlignment="Center">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<StackPanel Orientation="Horizontal" />
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
<ItemsControl.ItemTemplate>
<DataTemplate>
<StackPanel>
<Grid>
<Rectangle Height="100" Width="100" Margin="10">
<Rectangle.Fill>
<SolidColorBrush Color="{Binding Color}" />
</Rectangle.Fill>
<Interactivity:Interaction.Behaviors>
<Core:EventTriggerBehavior EventName="PointerPressed">
<Core:CallMethodAction MethodName="SelectMe" TargetObject="{Binding}"/>
</Core:EventTriggerBehavior>
</Interactivity:Interaction.Behaviors>
</Rectangle>
<TextBlock Text="X" VerticalAlignment="Top" HorizontalAlignment="Right" FontSize="50" Margin="20,10" Foreground="Wheat">
<Interactivity:Interaction.Behaviors>
<Core:EventTriggerBehavior EventName="PointerPressed">
<Core:CallMethodAction MethodName="RemoveMe" TargetObject="{Binding}"/>
</Core:EventTriggerBehavior>
</Interactivity:Interaction.Behaviors>
</TextBlock>
</Grid>
<Rectangle Height="10" Width="100" Margin="10"
Fill="White" Visibility="{Binding Selected}" />
</StackPanel>
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Grid>
Will look like this:
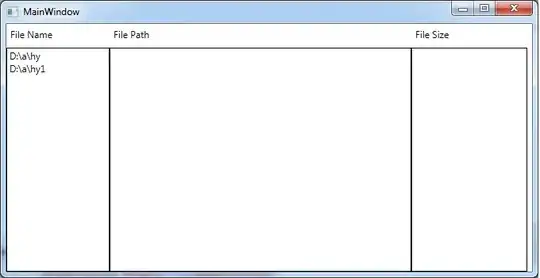
Best of luck!