My answer is a somewhat general solution for linear recurrence sequences. You would need some basic algebra knowledge in order to understand it completely.
Let we have a vector
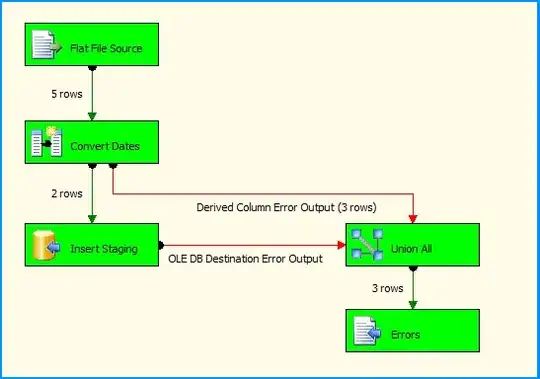
and we multiply it with the matrix
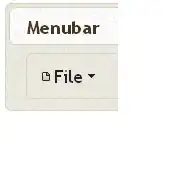
We would receive:
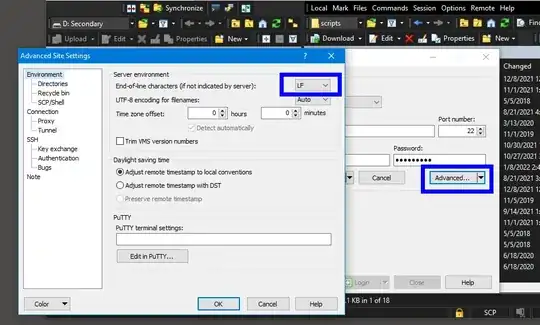
Therefore, when we multiply the vector with this matrix we receive the next fibonacci number. But what would happen if we multiply the vector with T2?
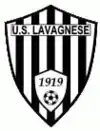
In this way, we constructed the fibonacci number after the next one (the (n+3)-th one). Now what would we get if we start with the first two fibonacci numbers in this vector and multiply it with Tn-1?

So by multiplying our vector with the matrix T, raised to the (n-1)th power, we can get the n-th fibonacci number. We can compute Tn-1 in time O(log n) via exponentiation by squaring. And of course, we should do all our calculations by modulo 108 + 7.
Here is a link of my implementation (in Java): http://pastie.org/8519742
This algorithm should work well and fast for all positive values of n up to 2108.
Sample running time of the method (using the same time measurement as in Peter Lawrey's answer):
fib(1,000,000,000,000,000,000) is 8,465,404 took us 1022.8 to calculate
fib(100,000,000,000,000,000) is 60,687,801 took us 325.7 to calculate
fib(10,000,000,000,000,000) is 9,115,009 took us 247.2 to calculate
fib(1,000,000,000,000,000) is 8,361,917 took us 233.3 to calculate
fib(100,000,000,000,000) is 11,279,600 took us 218.3 to calculate
fib(10,000,000,000,000) is 72,758,000 took us 6027.7 to calculate
fib(1,000,000,000,000) is 82,461,898 took us 184.2 to calculate
fib(100,000,000,000) is 60,584,292 took us 180.4 to calculate
fib(10,000,000,000) is 68,453,509 took us 162.0 to calculate
fib(1,000,000,000) is 90,703,191 took us 145.4 to calculate
fib(100,000,000) is 21 took us 131.3 to calculate
fib(10,000,000) is 60,722,758 took us 112.0 to calculate
fib(1,000,000) is 72,117,251 took us 99.8 to calculate
fib(100,000) is 33,178,829 took us 92.3 to calculate
fib(10,000) is 49,520,320 took us 70.8 to calculate
fib(1,000) is 95,802,669 took us 60.1 to calculate
fib(100) is 24,278,230 took us 39.3 to calculate
fib(10) is 55 took us 27.0 to calculate
fib(1) is 1 took us 16.3 to calculate
However, with all that said, this is not the fastest algorithm for your problem. It is well known that the fibonacci numbers have periodical residues under some module. Quoting the wikipedia entry on fibonacci numbers:
It may be seen that if the members of the Fibonacci sequence are taken mod n, the resulting sequence must be periodic with period at most n2−1.
In other words, if you find this period (with for example, the tortoise and hare algorithm - linear complexity), you can also find the result of every fibonacci number modulo 108+7.