There's some confusion.
- You can't have Spring MVC without servlets, simply because Spring MVC is built on top of the Servlet technology. (But that is not a problem, because you can have other view technologies.)
- You are confusing view technologies with servlets.
- There are several possible view technologies.
- If something, servlets are controllers, not views (actually, Spring MVC follows a Model 2 MVC, so servlets are not controllers in
the same sense as used in the standard MVC pattern).
The Spring Framework has a chapter dedicated to view technologies: http://docs.spring.io/spring/docs/3.2.x/spring-framework-reference/html/view.html
There you can find info and how to configure several views technologies, such as:
But don't be limited to that guide. There are other view technologies not listed there (as the view mechanism is very flexible and extensible). A very good example of one that is not listed there is Thymeleaf.
I'll try to clarify the Servlet vs. Controller question:
MVC Pattern
"Standard" MVC pattern works like the picture below:

The first source of confusion is to try to "fit" Spring MVC (because of the name) into this MVC. This usually leads to a mistake, as Spring MVC relates better to a variation of this pattern, as discussed below.
About the question, what I mean is: If really you just want to take this MVC pattern into account, Servlets are more like controllers, not views.
Model 2 MVC Pattern
To be really accurate, as said, Spring MVC does not follow the "standard" MVC, in fact it is more in tune with a Model 2 MVC, which includes a front controller component in addition to the already existing ones (model, view and controller), like the image:
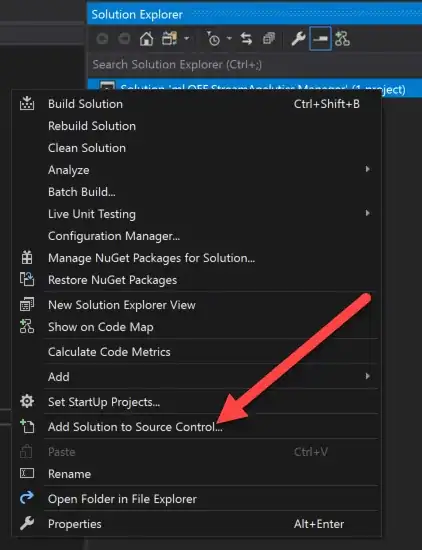
Now, in the Model 2 MVC, Servlets are not the controller components. They are the Front Controller (in fact, org.springframework.web.servlet.DispatcherServlet
is the front controller).
In this picture:
- Front Controller is the
org.springframework.web.servlet.DispatcherServlet
.
- Controllers are your classes (usually annotated with
@Controller
).
- View Template is a JSP/VM/HTML file, which will be rendered, based on the Model, by your selected View Technology (as listed before JSP, Tiles, Velocity, Thymeleaf... -- not Servlets).
- Model is the data (objects) from your domain, being passed around from the controller to the view.
What view engines are most commonly used?
This is a very relative question. All of those listed in the Spring reference are very popular. Aside from them, Thymeleaf is another very good I know.
It is not fair to say one is better than the other, and the ultimate decision would depend on your project's needs. Nevertheless, here's my two cents about the ones I've used:
- JSP is the oldest (which is good and bad at the same time) and one of the fastest, but can be very verbose and allow use of Java snippets in the pages.
- JSTL makes the syntax of JSP pages better, at the cost of performance.
- Freemarker and Velocity look very alike, they are very fast, their syntax is different than JSP (wether it is clearer is probably a personal opinion) and they have very good templating system.
- I haven't used Tiles, but I hear they have very good templating and componentization.
- Thymeleaf bets on having very maintainable HTML, and is a very healthy project with a growing comunity. See Thymeleaf vs. JSP.