I have recently created a demo and uploaded here.
It is using JodaTime
library for efficient results.
I hope it will be useful.
Screenshot:
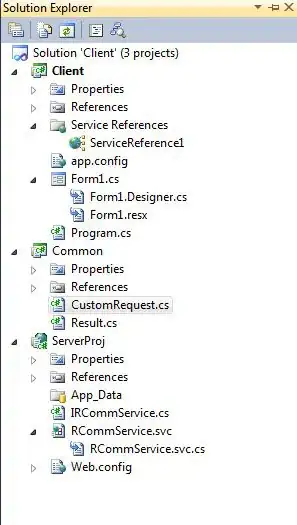
Code:
MainActivity.java
public class MainActivity extends Activity {
private SimpleDateFormat mSimpleDateFormat;
private PeriodFormatter mPeriodFormat;
private Date startDate;
private Date endDate;
private Date birthDate;
private TextView tvStartDate,tvEndDate,tvDifferenceStandard,tvDifferenceCustom,tvBirthDate,tvAgeStandard,tvAgeCustom;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
init();
//determine dateDiff
Period dateDiff = calcDiff(startDate,endDate);
tvDifferenceStandard.setText(PeriodFormat.wordBased().print(dateDiff));
tvDifferenceCustom.setText( mPeriodFormat.print(dateDiff));
//determine age
Period age = calcDiff(birthDate,new Date());
tvAgeStandard.setText(PeriodFormat.wordBased().print(age));
tvAgeCustom.setText( mPeriodFormat.print(age));
}
private void init() {
//ui
tvStartDate = (TextView)findViewById(R.id.tvStartDate);
tvEndDate = (TextView)findViewById(R.id.tvEndDate);
tvDifferenceStandard = (TextView)findViewById(R.id.tvDifferenceStandard);
tvDifferenceCustom = (TextView)findViewById(R.id.tvDifferenceCustom);
tvBirthDate = (TextView)findViewById(R.id.tvBirthDate);
tvAgeStandard = (TextView)findViewById(R.id.tvAgeStandard);
tvAgeCustom = (TextView)findViewById(R.id.tvAgeCustom);
//components
mSimpleDateFormat = new SimpleDateFormat("dd/MM/yy");
mPeriodFormat = new PeriodFormatterBuilder().appendYears().appendSuffix(" year(s) ").appendMonths().appendSuffix(" month(s) ").appendDays().appendSuffix(" day(s) ").printZeroNever().toFormatter();
try {
startDate = mSimpleDateFormat.parse(tvStartDate.getText().toString());
endDate = mSimpleDateFormat.parse(tvEndDate.getText().toString());
birthDate = mSimpleDateFormat.parse(tvBirthDate.getText().toString());
} catch (ParseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private Period calcDiff(Date startDate,Date endDate)
{
DateTime START_DT = (startDate==null)?null:new DateTime(startDate);
DateTime END_DT = (endDate==null)?null:new DateTime(endDate);
Period period = new Period(START_DT, END_DT);
return period;
}
}
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Date Diff Calculator"
android:textStyle="bold"
android:gravity="center"
android:background="@android:color/darker_gray"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="Start Date:" />
<TextView
android:id="@+id/tvStartDate"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="06/09/2011" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="End Date:" />
<TextView
android:id="@+id/tvEndDate"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="29/10/2013" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="Difference (Standard)" />
<TextView
android:id="@+id/tvDifferenceStandard"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="result" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="Difference (Custom)" />
<TextView
android:id="@+id/tvDifferenceCustom"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="result" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Age Calculator"
android:textStyle="bold"
android:gravity="center"
android:background="@android:color/darker_gray"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="Birth Date:" />
<TextView
android:id="@+id/tvBirthDate"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="01/09/1989" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="Age (Standard)" />
<TextView
android:id="@+id/tvAgeStandard"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="result" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold"
android:text="Age (Custom)" />
<TextView
android:id="@+id/tvAgeCustom"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="result" />
Note:
1)
don't forget to add JodaTime library
to your project
2)
As you can see in layout file, I have used fixed value for "Start Date","End Date" to calculate Date Difference
and fixed value for "Birth Date" to calculate Age
. You may replace it with your dynamic values
.