This will explain which one to use :)
Dim ws As Worksheet
Sub Sample()
Set ws = ThisWorkbook.Sheets("Sheet1")
Debug.Print foo1("B") '<~~ Col Name
Debug.Print foo2(Range("B1")) '<~~ Range
Debug.Print foo3(2) '<~~ Col Number
Debug.Print foo4("R1C2") '<~~ RC Notation
End Sub
Function foo1(ColRef As String) As Long
foo1 = ws.Cells(ws.Rows.Count, ColRef).End(xlUp).Row
End Function
Function foo2(ColRef As Range) As Long
foo2 = ws.Cells(ws.Rows.Count, ColRef.Column).End(xlUp).Row
End Function
Function foo3(ColRef As Integer) As Long
foo3 = ws.Cells(ws.Rows.Count, ColRef).End(xlUp).Row
End Function
Function foo4(ColRef As String) As Long
Dim MYAr
MYAr = Split(ColRef, "C", , vbTextCompare)
foo4 = ws.Cells(ws.Rows.Count, Val(MYAr(UBound(MYAr)))).End(xlUp).Row
End Function
Screenshot
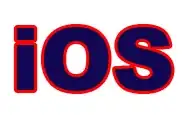
EDIT:
What is to be noted is that "C[-2]" is not Column B
it is an offset from the current cell. Here is another example which can handle different RC
notations. Note, I am not doing any error handling but I am sure you can handle that. The objective of this code is to show how to find the last row using RC
notation
Dim ws As Worksheet
Sub Sample()
Set ws = ThisWorkbook.Sheets("Sheet1")
Debug.Print foo4("C[-2]")
Debug.Print foo4("RC[-2]")
Debug.Print foo4("R[-1]C[-2]")
End Sub
Function foo4(ColRef As String) As Long
Dim MYAr, sTmp As String
Dim colNo As Integer
Dim rng As Range
'~~> I am assuming that you will pass a `C`. If not then
'~~> Add an error handling here
MYAr = Split(ColRef, "C", , vbTextCompare)
If InStr(1, MYAr(UBound(MYAr)), "[") Then
tmpAr = Split(MYAr(UBound(MYAr)), "[")(1)
tmpAr = Split(tmpAr, "]")(0)
colNo = Val(Trim(tmpAr))
Else
colNo = MYAr(UBound(MYAr))
End If
Set rng = ActiveCell.Offset(, colNo)
foo4 = ws.Cells(ws.Rows.Count, rng.Column).End(xlUp).Row
End Function