The random number generator is not truly random: say you seed it with 12 and make 100 random numbers, repeat the process and seed it with 12 again and make another 100 random numbers, they will be the same.
I have attached a small sample of 2 runs at 20 entries each with the seed of 12 to illustrate, immediately after the code which created them:
#include <iostream>
#include <cstdlib>
using namespace std;
int main()
{
srand(12);
for (int i =0;i<100; i++)
{
cout << rand() << endl;
}
return 0;
}
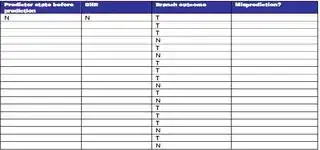
To avoid such repetition it is commonplace to use a more unique value, and as the time is always changing, and the chances of a two programs generating random sequences at exactly the same time are slim (especially when at the millisecond level), one can reasonably safely use time as an almost unique seed.
Requirements: seeding only need take place once per unique random sequence you need to generate.
However, there is an unexpected up-/down-side to this:
if the exact time is known of when the first sequence is generated then the exact sequence can be re-generated in the future by entering the seed value manually, causing the random number generator to step through its process in the same fashion as before (this is an upside for storing random sequences, and a downside for preserving their randomness).