I think you made a mistake in your query: look at the last two characters of the pattern - it clearly was unbalanced! Looks like you failed to copy-paste from PHP ;-)
- yours:
<div (id="(?<id>[a-zA-Z0-9]+)"|name="(?<name>[a-zA-Z0-9]+))"
- mine:
<div (id="(?<id>[a-zA-Z0-9]+)"|name="(?<name>[a-zA-Z0-9]+)")
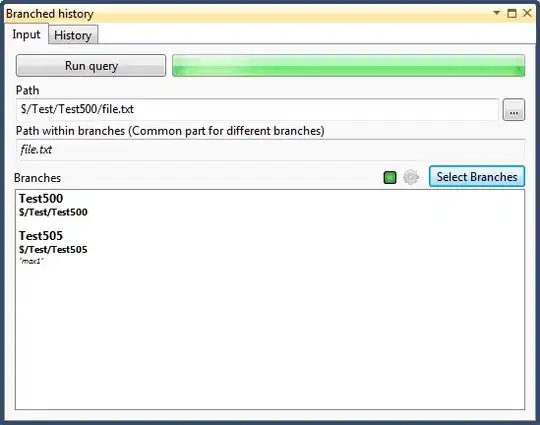
Using pcre.org engine + interactive editor from http://www.yunqa.de/delphi/doku.php/products/regex/index
I also tried this class: http://www.regular-expressions.info/delphi.html
That page immediately shows another interactive editor that could be used to debug your RegEx program: http://www.regexbuddy.com/test.html
I wonder why didn't you tried to use it...
Still i think some HTML parser would be both faster and more reliable. Consider HTML extracts like
<!-- <p><div name="bla-bla"> ... </div></p> -->
or like
<img src="...." alt='Press to insert <div id="123"> to you sample text' />
or like
<DIV ID="my cool id" />
The topic starter made his own answer below, consisting mostly of questions to me.
The problem is not the Regex,
Just count the quotes and arrows, in which order they are opened and in which they are closed, with pen and paper. You pattern is ( ... " ... ) .... "
- it is unbalanced!
is the Delphi.
Delphi the language does not have anything to do with regexps. The libraries/components can do. So that claim has no sense. You may argue that you tested broken libraries, but not the language itself.
My regex with PHP works fine,
That should mean that either you have different regex pattern in PHP (you did not copied here PHP source) or "Problem is in PHP"
Actually we did not saw neither Delphi source nor PHP source.
array[0] = '<div (id="(?<id>[a-zA-Z0-9]+)"|name="(?<name>[a-zA-Z0-9]+))"';
- is i think not correct line in neither.
So i don't think your code and patterns in PHP program and Delphi program match each other. Show quotes of the real code being used.
the thing is that DELPHI doesn't return me
- Again, that just does not makle sense. Delphi is just a language, it does not know a thing about RegEx.
- Just above you sawthe screenshot of Delphi-written program using PCRE engine - given the repaired pattern it DOES return both name and value. So the claim is obviously wrong even in vague sense. Delphi DOES return
<name, value>
pair for it.
Also, I can't change the whole system to use a HTML parser, the regex is already working
Then you need to adapt regex to correctly parse the HTML snippets i shown above.