Note: Works with "Lorem ipsum dolor dolor" as well.
Good evening everyone. I have figured out another technique. This one uses a varying approach to what Mark Baker did, which I appreciate very much. Also, go down to see memory usage.
In a nutshell, it takes basic string (lorem ipsum dolor) that needs to be matched, which is then shuffled into all possible combinations (in this case 3! = 6).
Further,all those 6 combination of strings are then added into array which is used to do the matching substr_count. I am also using shuffle()
, in_array
and array_push
.
The code is self explanatory and if you are curious, here's my IDEONE. This is Mark Baker's solution on IDEONE. They both take the same amount of time and memory, and my solution is 4 lines shorter, if not more elegant :)
<?php
$string = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ipsum lorem dolor Curabitur ac risus nunc. Dolor ipsum lorem.';
//convert main string to lowercase to have an even playing field
$string2 = strtolower($string);
$substring = 'lorem ipsum dolor';
//add the first lorem ipsum dolor to launch the array
$arr = array($substring);
//run until the array is full with all possible combinations i.e. 6 (factorial of 3)
for ($i=0; $i<=20; $i++) {
$wordArray = explode(" ",$substring);
shuffle($wordArray);
$randString= implode(" ",$wordArray);
//if random string isn't in the array, then only you push the new value
while (! (in_array($randString,$arr)) ) {
array_push($arr,$randString);
}
}
//var_dump($arr);
//here, we do the matching, and this is pretty self explanatory
$n = sizeof($arr);
for ($q=0; $q<=$n; $q++) {
$sum += substr_count($string2,$arr[$q]);
}
echo "Total occurances: ".$sum;
?>
Memory Usage
As you can already see, Mark's code ups me on +2 occasions, but the difference is very negligible due to the nature of this programme, and the data complexity associated. Obviously, the difference could be big given the program's complexity, but this is what it is.
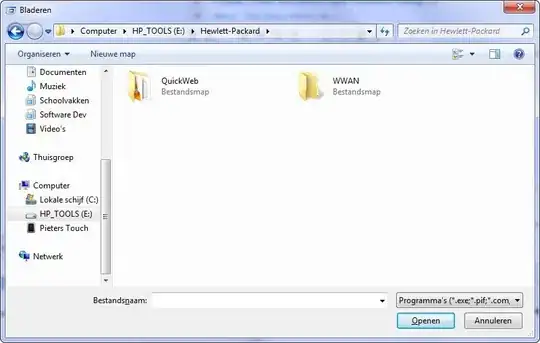