I have a class named BoardGUI extended from JFrame, in a constructor i
have made a JPanel with two buttons in it. I have added this panel
into my frame. Whenever i run this program, buttons get invisible. As
i bring my mouse cursor over the buttons they get visible.
setVisible(true);
should be last code line in constructor, because you added JComponents
to the already visible JFrame
,
or to call revalidate()
and repaint()
in the case that JComponents
are added to visible Swing GUI
there no reason to call a.setVisible(true);
or r.setVisible(true);
for standard JComponents
, because JComponents
are visible(true)
by default in comparing with Top Level Containers
, there you need to call JFrame/JDialog/JWindow.setVisible(true);
EDIT
(i used the very first suggestion you gave. problem remains the same.
) - for example
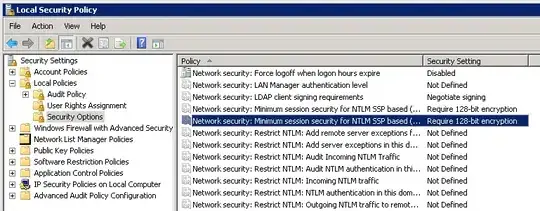
from code
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class MyGridLayout {
private JFrame frame = new JFrame("GridLayout, JButtons, etc... ");
private JPanel panel = new JPanel(new GridLayout(8, 8));
public MyGridLayout() {
for (int row = 0; row < 8; row++) {
for (int col = 0; col < 8; col++) {
JButton button = new JButton("(" + (row + 1) + " / " + (col + 1) + ")");
button.putClientProperty("column", col);
button.putClientProperty("row", row);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JButton btn = (JButton) e.getSource();
System.out.println(
"clicked column : "
+ btn.getClientProperty("column")
+ ", row : " + btn.getClientProperty("row"));
}
});
panel.add(button);
}
}
frame.add(panel);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocation(150, 150);
frame.setVisible(true);
}
public static void main(String[] args) {
javax.swing.SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
MyGridLayout myGridLayout = new MyGridLayout();
}
});
}
}