You can use PowerShell Jobs to run multiple robocopy jobs simultaneously. For more information, run:
Get-Help -Name about_Jobs;
Alternatively, you can simply call Start-Process
several times, and omit the -Wait
parameter.
Get-Help -Name Start-Process -Full;
Here is an example of how to kick off several PowerShell Background Jobs, and return status from all of them:
# Define a ScriptBlock that does some "work"
# NOTE: ScriptBlock should output an integer that indicates percentage complete
$ScriptBlock = {
1..100 | % { $_; Start-Sleep -Milliseconds (Get-Random -Minimum 5 -Maximum 200); };
}
# Kick off several jobs (with unique names)
1..3 | % { Start-Job -ScriptBlock $ScriptBlock -Name ('Complex Job {0}' -f $_); };
# Display Progress Bars until all jobs are completed
while (($JobList = Get-Job -State Running)) {
foreach ($Job in $JobList) {
try {
# Get the most recent status
$Percent = (Receive-Job -Job $Job -Keep)[-1];
Write-Progress -Activity 'Background Jobs' -CurrentOperation $Job.Name -Id $Job.Id -PercentComplete $Percent;
}
catch { Write-Verbose -Message ('Couldn''t get percentage completed from: {0}' -f $Job.Id); }
}
Start-Sleep -Milliseconds 200;
}
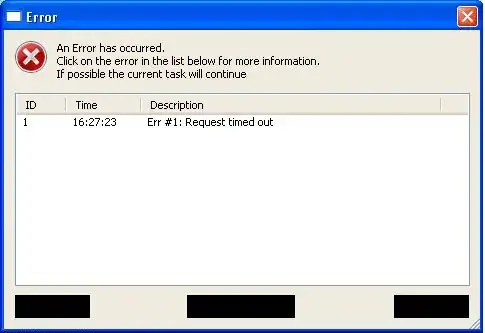