@Mark Redman certainly has the right idea:
Draw a gem-with-transparent-background over your metal setting.
This is what the gem images might look like:
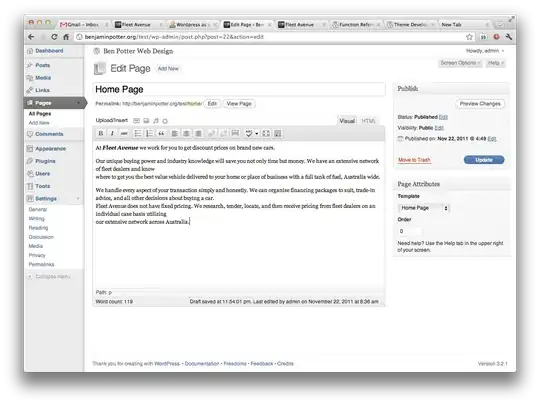
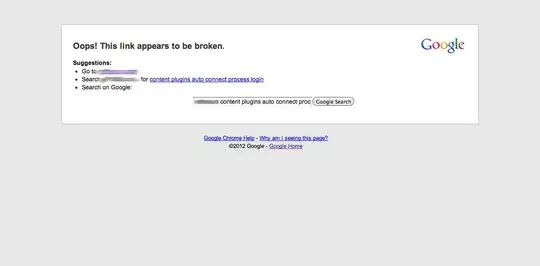
Demo of customer-selected gems drawn on top of a pendant setting: http://jsfiddle.net/m1erickson/U8Z2g/

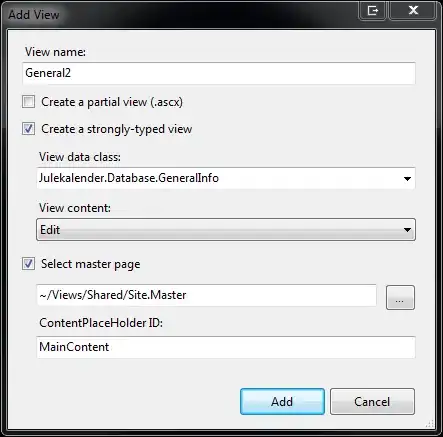
After the user has selected their gemstone, you can save the canvas to a .png image url like this:
var pngURL = canvas.toDataURL();
And here's what the code might look like:
<!doctype html>
<html>
<head>
<link rel="stylesheet" type="text/css" media="all" href="css/reset.css" /> <!-- reset css -->
<script type="text/javascript" src="http://code.jquery.com/jquery.min.js"></script>
<style>
body{ background-color: ivory; }
#canvas{border:1px solid red;}
button{display:none;}
</style>
<script>
$(function(){
var canvas=document.getElementById("canvas");
var ctx=canvas.getContext("2d");
// image loader
var imageURLs=[]; // put the paths to your images here
var imagesOK=0;
var imgs=[];
imageURLs.push("https://dl.dropboxusercontent.com/u/139992952/stack1/pendant.jpg");
imageURLs.push("https://dl.dropboxusercontent.com/u/139992952/stack1/purpleCZ.png");
imageURLs.push("https://dl.dropboxusercontent.com/u/139992952/stack1/pinkCZ.png");
loadAllImages();
function loadAllImages(callback){
for (var i=0; i<imageURLs.length; i++) {
var img = new Image();
imgs.push(img);
img.onload = function(){
imagesOK++;
if (imagesOK>=imageURLs.length ) {
$("button").show();
}
};
img.onerror=function(){alert("image load failed:"+this.src);}
img.crossOrigin="anonymous";
img.src = imageURLs[i];
}
}
$("#purple").click(function(){
draw(1);
});
$("#pink").click(function(){
draw(2);
});
function draw(index){
var stone=imgs[index];
ctx.clearRect(0,0,canvas.width,canvas.height);
ctx.drawImage(imgs[0],0,0,800,800,0,0,300,300);
ctx.drawImage(stone,0,0,stone.width,stone.height,108,134,stone.width*1.24,stone.height*1.24);
}
}); // end $(function(){});
</script>
</head>
<body>
<button id="purple">Purple</button>
<button id="pink">Pink</button><br>
<canvas id="canvas" width=300 height=300></canvas>
</body>
</html>