Solution 1: single-line, delete ,
Here you go with an SED one-liner:
sed -r 's/([^,],[^,],[^,],)(.*)(,.+,.+)/\1'"$(sed -r 's/([^,],[^,],[^,],)(.*)(,.+,.+)/\2/' <<< $myInput | sed 's/,//g')"'\3/' <<< $myInput
You have to replace <<< $myInput
with whatever your actual input is.
As you're working with CSVs you may have to tweak (both occurences of) the regex to match on each line of your CSV sheet.
In case your first three and last two fields are bigger than one char replace [^,]
with [^,]*
.
Explanation:
We use this regex
/([^,],[^,],[^,],)(.*)(,.+,.+)/
which captures the first (F,G,H,
), second (.*
) and last part (,D,E
) of the string for us.
The first and third capture group will be unchanged, while the second is going to be substitued.
For the substitution we call sed
a second (and actually third) time. First we capture only the second group, second we replace every ,
with nothing (only in the capture group!).
Proof:
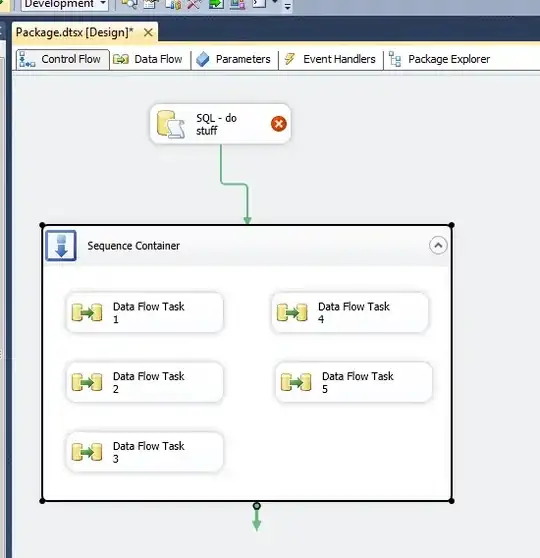
Of course, if there is no unwanted comma, nothing gets replaced:
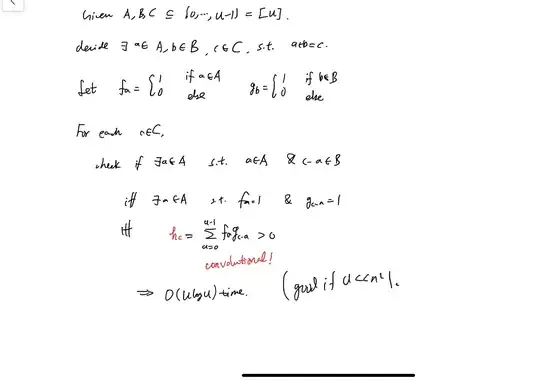
## Solution 2: whole file, line-by-line, delete `,` ##
If you want to specify only **a file** and the replacement should happen for each line of the file you can use
while read line; do sed -r 's/([^,],[^,],[^,],)(.*)(,.+,.+)/\1'"$(sed -r 's/([^,],[^,],[^,],)(.*)(,.+,.+)/\2/' <<< $line | sed 's/,//g')"'\3/' <<< $line; done < input.txt
where input.txt
at the end is - obviously - your file.
I just use the SED-command from above within a while
-loop which reads each line of the text. This is necessary because you have to keep track of the line you're reading, as you're calling sed
two times on the same input.
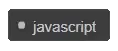
## Solution 3: whole file, enclose field in `"` ##
As [@Łukasz L.][4] pointed out in the comments to the OP, according to the [RFC1480][5], which describes the format for CSV-files it would be better to enclose fields which contain a comma in `"`.
This is more simple than the other solutions:
sed -r 's/([^,],[^,],[^,],)(.*)(,.*,.*)/\1"\2"\3/' input.txt
Again we have the three capturing groups. This allows us to simply wrap the second group in "
!
