For you there are many approach. Once such Simple is this :
- Add a view to your keyWindow & keep it hidden. This view should contain all your message & button too. Do this in AppDelegate.
Provide this code in
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
UIView *view = [[UIView alloc]initWithFrame:self.window.frame];
[view setBackgroundColor:[UIColor colorWithWhite:0.2 alpha:0.7]];
UIButton * loginButton = [UIButton buttonWithType:UIButtonTypeCustom];
[loginButton setTitle:@"Sign In" forState:UIControlStateNormal];
[loginButton setTitleColor:[UIColor blueColor] forState:UIControlStateNormal];
[loginButton setBackgroundImage:GETIMAGE(@"loginNormal", @"png") forState:UIControlStateNormal];
[loginButton setBackgroundImage:GETIMAGE(@"loginPressed", @"png") forState:UIControlStateHighlighted];
[loginButton setFrame:CGRectMake(100, 300, 100, 40)];
[loginButton addTarget:self action:@selector(LoginMe:) forControlEvents:UIControlEventTouchUpInside];
[view addSubview:loginButton];
UIButton * cancelButton = [UIButton buttonWithType:UIButtonTypeCustom];
[cancelButton setTitleColor:[UIColor redColor] forState:UIControlStateNormal];
[cancelButton setTitle:@"Cancel" forState:UIControlStateNormal];
[cancelButton setBackgroundImage:GETIMAGE(@"loginNormal", @"png") forState:UIControlStateNormal];
[cancelButton setBackgroundImage:GETIMAGE(@"loginPressed", @"png") forState:UIControlStateHighlighted];
[cancelButton setFrame:CGRectMake(100, 350, 100, 40)];
[cancelButton addTarget:self action:@selector(DismissMe:) forControlEvents:UIControlEventTouchUpInside];
[view addSubview:cancelButton];
[[[UIApplication sharedApplication] keyWindow] addSubview:view];
view.center = [[UIApplication sharedApplication] keyWindow].center;
[view setTag:666666];
[view setAlpha:0.0];
- Implement your methods
DismissMe
and LoginMe
- Now On the click on some thing as you said *when he clicks on messages or my page *
Add this code if user is unauthorized.
[[[UIApplication sharedApplication] keyWindow] bringSubviewToFront:[[[UIApplication sharedApplication] keyWindow] viewWithTag:666666]];
[[[[UIApplication sharedApplication] keyWindow] viewWithTag:666666] setAlpha:1.0];
Now in LoginMe implementation, you can do something like this Modal.
UIViewController *topController = [UIApplication sharedApplication].keyWindow.rootViewController;
if([self.window.rootViewController isKindOfClass:[UITabBarController class]]) {
self.loginController = [[MyLoginViewController alloc]init];
[topController presentViewController:self.loginController animated:YES completion:nil];
}
here is some screenshot:
- My home Screen
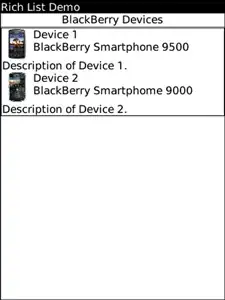
- SignIn Cancel if Unauthorised.

I hope that helps.