The problem here is that you're not utilizing a group element to create a layer to transition. As you'll see in your code, it is necessary to first bind the transition object to the svg layer, and then give the svg layer yet another layer (a element in this instance) to work with.
Use your D.O.M. inspector to view the children of the svg layer that you've appended.
You'll see that before you pan, the translation values of the g object are the default property values (translate(0,0).
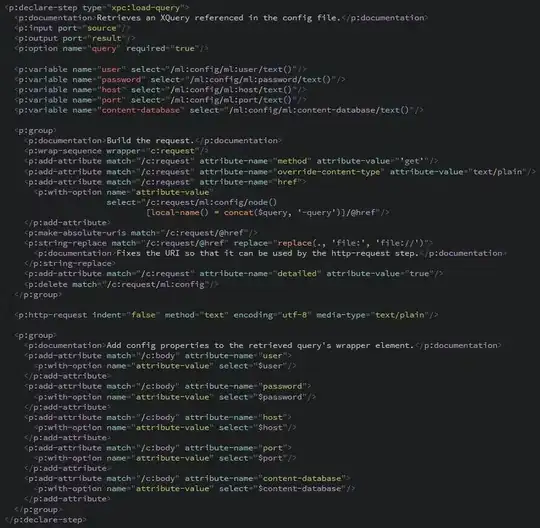
After you pan the translation attributes will be different.
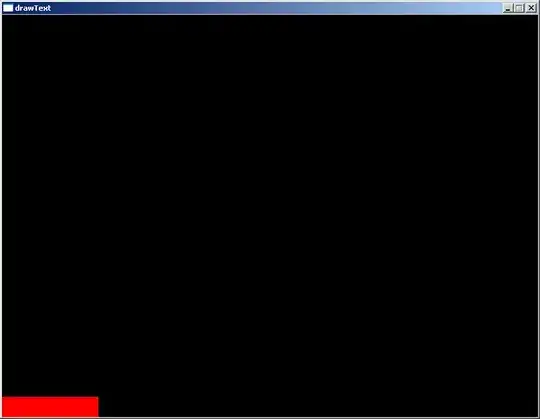
Essentially what is happening is that the g layer is being used as the main layer to append items to. The svg layer in turn is acting as sort of a mask/window to view the translated g layer. This approach will also work with svg elements in exchange for g elements.
Here is a jsfiddle for the corresponding code below: http://jsfiddle.net/blakedietz/seU2y/
Please note that I had to make quite a few modifications to get your code to work. For future reference if you want someone to answer your question more swiftly I would advise that you create some sort of working example of your code. Toy examples with fake data are often the best way to convey your problem at hand without causing the reader to do too much guess work to figure out what it is that you're trying to communicate.
// ********* START MY CODE
var width = 500;
var height = 500;
var margin = {top:0, right:0,bottom:0,left:0};
var data = d3.range(1,20).map(function(datum)
{
var coordinates = {};
coordinates.xcord = datum;
coordinates.ycord = datum;
return coordinates;
});
var xDomain = d3.extent(data.map(function(datum){ return datum.xcord}));
var yDomain = d3.extent(data.map(function(datum){ return datum.ycord}));
var x = d3.scale.linear().domain(xDomain).range([margin.left, width - margin.right]);
var y = d3.scale.linear().domain(yDomain).range([height - margin.bottom, margin.top]);
function zoom()
{
svg.attr("transform", "translate(" + d3.event.translate + ")scale(" + d3.event.scale + ")");
}
// ********* END MY CODE
// ********* START YOUR CODE
var svg = d3.select("body").append("svg")
.attr("width", width + margin.left + margin.right)
.attr("height", height + margin.top + margin.bottom)
.call(d3.behavior.zoom().x(x).y(y).scaleExtent([1, 15]).on("zoom", zoom))
// MY MODIFICATION Adds g layer to append to.
.append("g")
.attr("transform", "translate(" + margin.left + "," + margin.top + ")");
// Compute the scales’ domains.
x.domain(d3.extent(data, function (d) { return d.xcord; })).nice();
y.domain(d3.extent(data, function (d) { return d.ycord; })).nice();
// Add the points!
svg.selectAll("circle")
.data(data)
.enter()
.append("circle")
//.attr("fill","grey")
.attr("cx", function (d) {
return x(d.xcord);
})
.attr("cy", function (d) {
return y(d.ycord);
})
.attr("r", 1);