I wrote about this in my open-source e-book. It has a section about object restrictions https://github.com/carltheperson/advanced-js-objects/blob/main/chapters/chapter-3.md#object-restrictions
To summarize:
This table shows the hierarchy of restrictions:
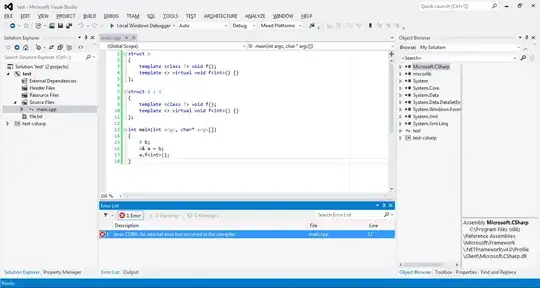
Object.preventExtensions
fail example
const obj = { a: "A", b: "B" }
Object.preventExtensions(obj)
obj.c = "C" // Failure
console.log(obj) // { a: "A", b: "B" }
Object.seal
fail example
const obj = { a: "A", b: "B" }
Object.seal(obj)
delete obj.a // Failure
obj.c = "C" // Failure
console.log(obj) // { a: "A", b: "B" }
Object.freeze
fail example
const obj = { a: "A", b: "B" }
Object.freeze(obj)
delete obj.a // Failure
obj.b = "B2" // Failure
obj.c = "C" // Failure
console.log(obj) // { a: "A", b: "B" }
Note: How they "fail" depends if your code is running in strict mode or not.
- Strict mode = error
- Not in strict mode = silent fail