You can record and play audio using the AVFoundation
framework. Firstly you will need to implement this within your .h
file and add a framework or library's within your xcode project settings like so:
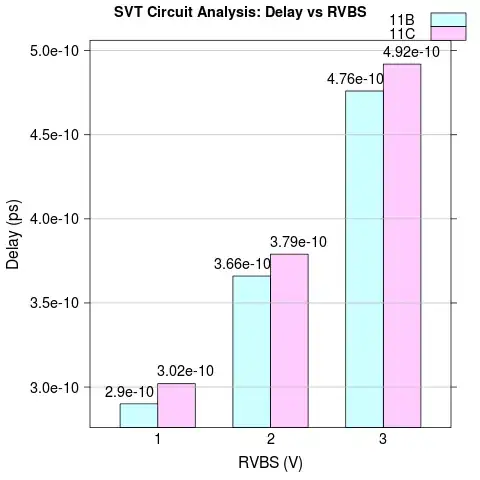
After adding into your project settings import AVFoundation
into your .h
file like so:
#import <AVFoundation/AVFoundation.h>
Now Implement your delegates within your .h
file:
@interface ViewController : UIViewController <AVAudioRecorderDelegate, AVAudioPlayerDelegate>
After this declare your AVAudioRecorder
and AVAudioPlayer
in your .h
file like so:
@interface ViewController () {
AVAudioRecorder *recorder;
AVAudioPlayer *player;
IBOutlet UIButton *stopButton;
IBOutlet UIButton *playButton ;
}
- (IBAction)recordPauseTapped:(id)sender;
- (IBAction)stopTapped:(id)sender;
- (IBAction)playTapped:(id)sender;
Now set up everything in the -(Void)ViewDidLoad{}
:
- (void)viewDidLoad
{
[super viewDidLoad];
// Disable Stop/Play button when application launches
[stopButton setEnabled:NO];
[playButton setEnabled:NO];
// Set the audio file
NSArray *pathComponents = [NSArray arrayWithObjects:
[NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES) lastObject],
@"MyAudioMemo.m4a",
nil];
NSURL *outputFileURL = [NSURL fileURLWithPathComponents:pathComponents];
// Setup audio session
AVAudioSession *session = [AVAudioSession sharedInstance];
[session setCategory:AVAudioSessionCategoryPlayAndRecord error:nil];
// Define the recorder setting
NSMutableDictionary *recordSetting = [[NSMutableDictionary alloc] init];
[recordSetting setValue:[NSNumber numberWithInt:kAudioFormatMPEG4AAC] forKey:AVFormatIDKey];
[recordSetting setValue:[NSNumber numberWithFloat:44100.0] forKey:AVSampleRateKey];
[recordSetting setValue:[NSNumber numberWithInt: 2] forKey:AVNumberOfChannelsKey];
// Initiate and prepare the recorder
recorder = [[AVAudioRecorder alloc] initWithURL:outputFileURL settings:recordSetting error:NULL];
recorder.delegate = self;
recorder.meteringEnabled = YES;
[recorder prepareToRecord];
}
Now Implement the recording Button Like so...
- (IBAction)recordPauseTapped:(id)sender {
// Stop the audio player before recording
if (player.playing) {
[player stop];
}
if (!recorder.recording) {
AVAudioSession *session = [AVAudioSession sharedInstance];
[session setActive:YES error:nil];
// Start recording
[recorder record];
[recordPauseButton setTitle:@"Pause" forState:UIControlStateNormal];
} else {
// Pause recording
[recorder pause];
[recordPauseButton setTitle:@"Record" forState:UIControlStateNormal];
}
[stopButton setEnabled:YES];
[playButton setEnabled:NO];
}
Now Implement the StopButton
IBAction:
- (IBAction)stopTapped:(id)sender {
[recorder stop];
AVAudioSession *audioSession = [AVAudioSession sharedInstance];
[audioSession setActive:NO error:nil];
}
Next Implement the playTapped
IBAction like so:
- (IBAction)playTapped:(id)sender {
if (!recorder.recording){
player = [[AVAudioPlayer alloc] initWithContentsOfURL:recorder.url error:nil];
[player setDelegate:self];
[player play];
}
}
Lastly Implement the required AVPlayer Delegate
by doing this:
- (IBAction)playTapped:(id)sender {
if (!recorder.recording){
player = [[AVAudioPlayer alloc] initWithContentsOfURL:recorder.url error:nil];
[player setDelegate:self];
[player play];
}
}
And that's it! The Finished Product should look something like this...
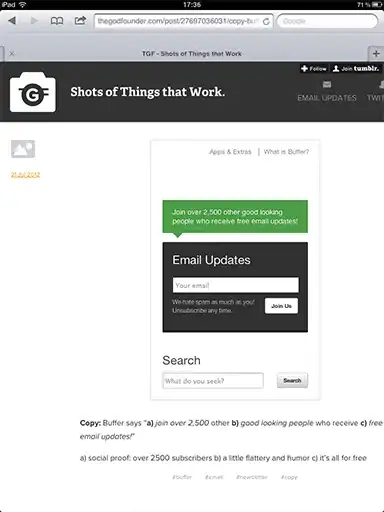
For more info take a look at These Links:
Link1
Link2
Link3
Documentation Links:
Link 1
Hope This Helps.