The official Javadoc (for JavaFX 8, but the logic remains unchanged in newer versions) says:
Bounding Rectangles
[...]
Each Node has a read-only boundsInLocal variable which specifies the bounding rectangle of the Node in untransformed local coordinates.
Each Node also has a read-only boundsInParent variable which specifies the bounding rectangle of the Node after all transformations have been applied, including those set in transforms, scaleX/scaleY, rotate, translateX/translateY, and layoutX/layoutY. It is called "boundsInParent" because the rectangle will be relative to the parent's coordinate system.
The behavior of the methods is the same when a Node is placed in an AnchorPane or a StackPane. The observed differences are because of the default layout of the AnchorPane (which places children in its top left corner) and the StackPane (which centers children).
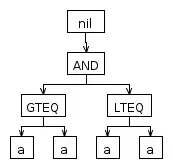
In this example picture, there are three black rectangles.
All rectangles are defined as:
[x=0.0, y=0.0, width=20.0, height=30.0, fill=0x000000ff]
For all three rectangles, getBoundsInLocal
is
[**minX:0.0, minY:0.0,** minZ:0.0, **width:20.0, height:30.0**, depth:0.0, maxX:20.0, maxY:30.0, maxZ:0.0]
The left parent (yellow) is an AnchorPane with the default anchors. It places the rectangle in its top left corner (coordinate 0,0). As there are no other transformations (scale, rotation, ...), the boundsInParent for the rectangle in the yellow AnchorPane are therefore identical to its boundsInLocal:
rect1.getBoundsInParent (AnchorPane yellow)=BoundingBox [minX:0.0, minY:0.0, minZ:0.0, width:20.0, height:30.0, depth:0.0, maxX:20.0, maxY:30.0, maxZ:0.0]
The center parent (green) is a StackPane, which centers its children by default. The x/y values of the contained rectangle's boundsInParent are therefore different from the boundsInLocal:
rect2.getBoundsInParent (StackPane green)=BoundingBox [minX:24.0, minY:35.20000076293945, minZ:0.0, width:20.0, height:29.999996185302734, depth:0.0, maxX:44.0, maxY:65.19999694824219, maxZ:0.0]
The right parent (red) is another AnchorPane, but with different anchors:
AnchorPane.setRightAnchor(rect3, 0d);
AnchorPane.setBottomAnchor(rect3, 0d);
Again, x/y values of the contained rectangle's boundsInParent are therefore different from the boundsInLocal:
rect3.getBoundsInParent (AnchorPane red)=BoundingBox [minX:47.20000076293945, minY:69.5999984741211, minZ:0.0, width:19.999996185302734, height:30.0, depth:0.0, maxX:67.19999694824219, maxY:99.5999984741211, maxZ:0.0]
This should show that there is no difference between the behavior/computation of the bounds in AnchorPane and StackPane - the difference is simply due to their default layouting, and is consistent in all cases.
To try for yourself, I here is the example code:
public class LocalAndParentBoundsApp extends Application {
public static void main(String[] args) {
LocalAndParentBoundsApp.launch(args);
}
@Override
public void start(Stage primaryStage) {
final Rectangle rect1 = new Rectangle(20, 30);
final Rectangle rect2 = new Rectangle(20, 30);
final Rectangle rect3 = new Rectangle(20, 30);
final AnchorPane anchorPane = new AnchorPane(rect1);
anchorPane.setPrefWidth(100);
anchorPane.setPrefHeight(100);
anchorPane.setStyle("-fx-background-color: yellow;");
final StackPane stackPane = new StackPane(rect2);
stackPane.setPrefWidth(100);
stackPane.setPrefHeight(100);
stackPane.setStyle("-fx-background-color: green;");
final AnchorPane anchorPane2 = new AnchorPane(rect3);
anchorPane2.setPrefWidth(100);
anchorPane2.setPrefHeight(100);
anchorPane2.setStyle("-fx-background-color: red;");
AnchorPane.setRightAnchor(rect3, 0d);
AnchorPane.setBottomAnchor(rect3, 0d);
primaryStage.setTitle("LocalAndParentBounds");
HBox root = new HBox(anchorPane, stackPane, anchorPane2);
primaryStage.setScene(new Scene(root, 200, 100));
primaryStage.show();
System.out.println("rect1=" + rect1);
System.out.println("rect1.getBoundsInLocal=" + rect1.getBoundsInLocal());
System.out.println("rect1.getBoundsInParent (AnchorPane yellow)=" + rect1.getBoundsInParent());
System.out.println("rect2=" + rect2);
System.out.println("rect2.getBoundsInLocal=" + rect2.getBoundsInLocal());
System.out.println("rect2.getBoundsInParent (StackPane green)=" + rect2.getBoundsInParent());
System.out.println("rect3=" + rect3);
System.out.println("rect3.getBoundsInLocal=" + rect3.getBoundsInLocal());
System.out.println("rect3.getBoundsInParent (AnchorPane red)=" + rect3.getBoundsInParent());
}
}