Here goes my solution. This is a working sample which will traverse the same user model between steps. It uses Model binding concept. More refinement to code is required, with proper validations, Datatime handling etc. At the end of last step, you should see completed filled model, which you can save it.
Model -
public class User
{
public string Fname { get; set; }
public string Lname { get; set; }
public List<AvailabilityDates> Dates { get; set; }
}
public class AvailabilityDates
{
public DateTime? date { get; set; }
}
Controller Actions -
public class UserController : Controller
{
public ActionResult Index()
{
User u = new User();
return View(u);
}
public ActionResult FirstStep(User u)
{
u.Dates = new List<AvailabilityDates>();
u.Dates.Add(new AvailabilityDates() { date = null });
u.Dates.Add(new AvailabilityDates() { date = null });
return View(u);
}
public ActionResult LastStep(User u)
{
// Do your stuff here
return null;
}
}
Index view -
@model MVC.Controllers.User
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
@using (Html.BeginForm("FirstStep", "User", FormMethod.Post))
{
@Html.LabelFor(model => model.Fname, new { @class = "control-label col-md-2" })
@Html.EditorFor(model => model.Fname)
@Html.ValidationMessageFor(model => model.Fname)
@Html.LabelFor(model => model.Lname, new { @class = "control-label col-md-2" })
@Html.EditorFor(model => model.Lname)
@Html.ValidationMessageFor(model => model.Lname)
<input type="submit" value="Create" class="btn btn-default" />
}
FirstStep View -
@model MVC.Controllers.User
@{
ViewBag.Title = "FirstStep";
}
<h2>FirstStep</h2>
@using (Html.BeginForm("LastStep", "User", FormMethod.Post))
{
@Html.HiddenFor(model => model.Fname);
@Html.HiddenFor(model => model.Lname);
for (int i = 0; i < Model.Dates.Count; i++)
{
@Html.LabelFor(model => Model.Dates[i].date, new { @class = "control-label col-md-2" })
@Html.EditorFor(model => Model.Dates[i].date)
@Html.ValidationMessageFor(model => Model.Dates[i].date)
}
<input type="submit" value="Create" class="btn btn-default" />
}
Output -
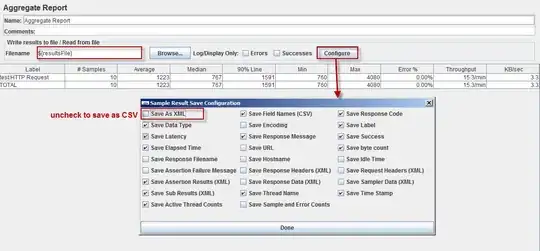
I would also suggest you to look into some JQuery good wizard controls -