The another way is to read all occurring CONST_*.XYZ
into FileListBox and then show the last.
procedure TForm1.Button1Click(Sender: TObject);
begin
FileListBox1.Directory:='D:\samples';
FileListBox1.Mask:='CONST_*.XYZ';
FileListBox1.Update;
Label1.Caption:= FileListBox1.Items[FileListBox1.Items.Count-1];
end;
To make it faster, you can use a function
function getRegion(filestr:string):Boolean;
begin
if FindFirst(filestr, faAnyFile, searchResult) = 0 then result:=true else result:=false;
if result then begin
findN:=filestr;
end;
end;
begin
SetCurrentDir('D:\samples');
for i:=9 downto 0 do begin
if getRegion(Format ('CONST_%.1d*.XYZ', [i])) then break;
end;
FileListBox1.Directory:='D:\samples';
FileListBox1.Mask:=findN;
FileListBox1.Update;
Label1.Caption:= FileListBox1.Items[FileListBox1.Items.Count-1];
Update
For test A) files were created from 0000-4999
For test B) files were created from 0000-9999
TestA made files from 0000
to only 4999
because user jpfollenius
uses downto
from 0000
to 4999
= 5000 files
from 9999
downto 4999
= 5000 files

Testtable TestA
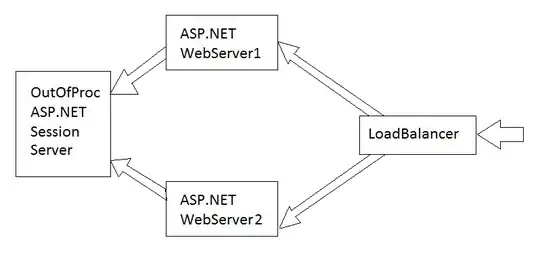
Test B
I'm shure with more files 50000 files my solution loads 10000 filenames
for example 50000
to 59999
that takes
- moskito-x .................................. 0.345 seconds (tested)
- pure FindFirst / FindNext .......... 0.390 seconds estimated to (0.039 * 10)