If the area is always a rectangle, the easiest way is to compare the coordinates.
Let's assume that your rectangle is defined by its upper left (r1x: lon and r1y: lat) and lower right (r2x and r2y) corners. Your object (a point) is defined by px: lon and py: lat.
So, your object is inside the area if
px > r1x and px < r2x
and
py < r1y and py > r2y
Programatically it would be something like:
boolean isPInR(double px, double py, double r1x, double r1y, double r2x, double r2y){
if(px > r1x && px < r2x && py < r1y && py > r2y){
//It is inside
return true;
}
return false;
}
EDIT
In the case where your polygon is not a rectangle, you can use the Java.awt.Polygon Class. In this class you will find the method contains(x,y) which return true if the point with x and y coordinates is inside the Polygon.
This method uses the Ray-casting algorithm. To simplify, this algorithm draw a segment in a random direction from your point. If the segment cross your polygon's boarder an odd number of times, then it is inside your polygon. If it crosses it an even number of times, then it is outside.
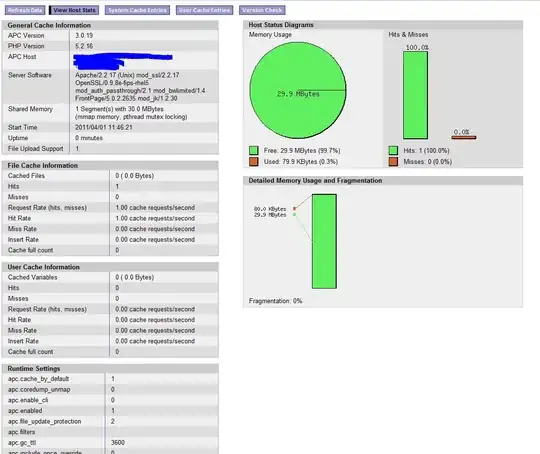
To use the polygon Class, you can do something like:
//This defines your polygon
int xCoord[] = {1,2,3,5,9,-5};
int yCoord[] = {18,-32,1,100,-100,0};
myPolygon = new Polygon(xCoord, yCoord, xCoord.length);
//This finds if the points defined by x and y coordinates is inside the polygon
Boolean isInside = myPolygon.contains(x,y);
And don't forget to
import java.awt.Polygon;
EDIT
Right coordinates are in Double !
So you need to use Path2D.Double !
Begin by import java.awt.geom.Path2D;
Let's say you start with similar arrays as before:
//This defines your polygon
Double xCoord[] = {1.00121,2,3.5464,5,9,-5};
Double yCoord[] = {18.147,-32,1,100,-100.32,0};
Path2D myPolygon = new Path2D.Double();
//Here you append all of your points to the polygon
for(int i = 0; i < xCoord.length; i++) {
myPolygon.moveTo(xCoord[i], yCoord[i]);
}
myPolygon.closePath();
//Now we want to know if the point x, y is inside the Polygon:
Double x; //The x coord
Double y; //The y coord
Boolean isInside = myPolygon.contains(x,y);
And here you go with Double !