UPDATED to eliminate the extra space in the output (changed (.+)
to [SPACE](.+)
).
Assuming each car is on a separate line, this works:
^(\d+ )(?:Blue|Red|Purple|Inferno Red) (.+)$
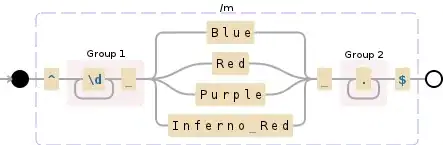
Debuggex Demo
Use
sNumColorCarLine.replaceAll(sTheRegex, "$1$2");
or
sNumColorCarLine.replaceFirst(sTheRegex, "$1$2");
to make the replacement. To be efficient though, especially if there are a lot of data lines, use the following, which avoids re-compiling the pattern (and re-creating the matcher) for every line:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
/**
<P>{@code java RemoveColorFromCarLinesNoLoops}</P>
**/
public class RemoveColorFromCarLinesNoLoops {
public static final void main(String[] igno_red) {
//Add colors as necessary
String sColorsNonCaptureOr = "(?:Blue|Red|Purple|Inferno Red)";
String sRegex = "" +
"^(\\d+ )" + //one-or-more digits, then one space
sColorsNonCaptureOr + //color
" (.+)$"; //Everything after the color (space uncaptured)
String sRplcWith = "$1$2";
//"": Unused search-string, so matcher can be reused.
Matcher m = Pattern.compile(sRegex).matcher("");
String sColorRemoved1 = removeColorFromCarLine(m, "04 Blue Honda Accord", sRplcWith);
String sColorRemoved2 = removeColorFromCarLine(m, "12 Inferno Red Chevrolet Tahoe", sRplcWith);
String sColorRemoved3 = removeColorFromCarLine(m, "10 Purple Ford Taurus", sRplcWith);
}
private static final String removeColorFromCarLine(Matcher m_m, String s_origCarLine, String s_rplcWith) {
m_m.reset(s_origCarLine);
if(!m_m.matches()) {
throw new IllegalArgumentException("Does not match: \"" + " + s_origCarLine + " + "\", pattern=[" + m_m.pattern() + "]");
}
//Since it matches(s), this is equivalent to "replace the entire line, as a whole"
String s = m_m.replaceFirst(s_rplcWith);
System.out.println(s_origCarLine + " --> " + s);
return s;
}
}
Output
[C:\java_code\]java RemoveColorFromCarLinesNoLoops
04 Blue Honda Accord --> 04 Honda Accord
12 Inferno Red Chevrolet Tahoe --> 12 Chevrolet Tahoe
10 Purple Ford Taurus --> 10 Ford Taurus