Here goes the solution -
Credit goes to this extraordinary gentleman - ThumNet, who wrote RadioButtonList for Enum as an extension
Step 1 - Create RadioButtonListEnum.cshtml file with below code (code from above reference) in Views/Shared/EditorTemplates directory (if not exist, then create that directory) -
@model Enum
@{
// Looks for a [Display(Name="Some Name")] or a [Display(Name="Some Name", ResourceType=typeof(ResourceFile)] Attribute on your enum
Func<Enum, string> getDescription = en =>
{
Type type = en.GetType();
System.Reflection.MemberInfo[] memInfo = type.GetMember(en.ToString());
if (memInfo != null && memInfo.Length > 0)
{
object[] attrs = memInfo[0].GetCustomAttributes(typeof(System.ComponentModel.DataAnnotations.DisplayAttribute),
false);
if (attrs != null && attrs.Length > 0)
return ((System.ComponentModel.DataAnnotations.DisplayAttribute)attrs[0]).GetName();
}
return en.ToString();
};
var listItems = Enum.GetValues(Model.GetType()).OfType<Enum>().Select(e =>
new SelectListItem()
{
Text = getDescription(e),
Value = e.ToString(),
Selected = e.Equals(Model)
});
string prefix = ViewData.TemplateInfo.HtmlFieldPrefix;
int index = 0;
ViewData.TemplateInfo.HtmlFieldPrefix = string.Empty;
foreach (var li in listItems)
{
string fieldName = string.Format(System.Globalization.CultureInfo.InvariantCulture, "{0}_{1}", prefix, index++);
<div class="editor-radio">
@Html.RadioButton(prefix, li.Value, li.Selected, new { @id = fieldName })
@Html.Label(fieldName, li.Text)
</div>
}
ViewData.TemplateInfo.HtmlFieldPrefix = prefix;
}
Then have your enum -
public enum QuestionEnd
{
[Display(Name = "Cancel Invitation")]
CancelInvitation,
[Display(Name = "Plan with participants on first available common date")]
FirstAvailableCommon,
[Display(Name = "Plan with participants on my first available common date")]
YourFirstAvailableCommon
}
Step 2 - Create Model -
public class RadioEnumModel
{
public QuestionEnd qEnd { get; set; }
}
Step 3 - Create Controller Action -
public ActionResult Index()
{
RadioEnumModel m = new RadioEnumModel();
return View(m);
}
Step 4 - Create View -
@model MVC.Controllers.RadioEnumModel
@Html.EditorFor(x => x.qEnd, "RadioButtonListEnum")
Then the output would be -
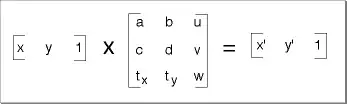