The answer depends on the definition of a "line". For example, if you are using a wrapped JTextArea
, where a single, contiguous line of text, wraps around the view, a line could be considered the text that runs from one side of the view to the other.
In this case you need to delve into the model and calculate the offsets of the text based on the view and basically remove the content between two points, for example...
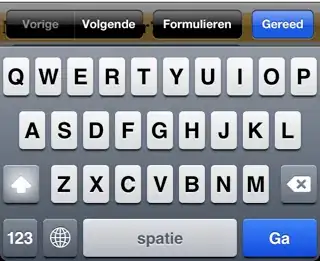
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import javax.swing.text.Element;
import javax.swing.text.JTextComponent;
import javax.swing.text.Utilities;
public class TestDeleteLine {
public static void main(String[] args) {
new TestDeleteLine();
}
private JTextArea ta;
public TestDeleteLine() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
ta = new JTextArea(20, 40);
ta.setWrapStyleWord(true);
ta.setLineWrap(true);
JButton deleteLine = new JButton("Delete current line");
deleteLine.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
int offset = ta.getCaretPosition();
int rowStart = Utilities.getRowStart(ta, offset);
int rowEnd = Utilities.getRowEnd(ta, offset);
Document document = ta.getDocument();
int len = rowEnd - rowStart + 1;
if (rowStart + len > document.getLength()) {
len--;
}
String text = document.getText(rowStart, len);
document.remove(rowStart, len);
} catch (BadLocationException ex) {
ex.printStackTrace();
}
}
});
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new JScrollPane(ta));
frame.add(deleteLine, BorderLayout.SOUTH);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
}
Now, if you don't care about the line wrapping and simple want to remove the entire line (from one new line to another) you could use...
public int getLineByOffset(int offset) throws BadLocationException {
Document doc = ta.getDocument();
if (offset < 0) {
throw new BadLocationException("Can't translate offset to line", -1);
} else if (offset > doc.getLength()) {
throw new BadLocationException("Can't translate offset to line", doc.getLength() + 1);
} else {
Element map = doc.getDefaultRootElement();
return map.getElementIndex(offset);
}
}
public int getLineStartOffset(int line) throws BadLocationException {
Element map = ta.getDocument().getDefaultRootElement();
if (line < 0) {
throw new BadLocationException("Negative line", -1);
} else if (line >= map.getElementCount()) {
throw new BadLocationException("No such line", ta.getDocument().getLength() + 1);
} else {
Element lineElem = map.getElement(line);
return lineElem.getStartOffset();
}
}
public int getLineEndOffset(int line) throws BadLocationException {
Element map = ta.getDocument().getDefaultRootElement();
if (line < 0) {
throw new BadLocationException("Negative line", -1);
} else if (line >= map.getElementCount()) {
throw new BadLocationException("No such line", ta.getDocument().getLength() + 1);
} else {
Element lineElem = map.getElement(line);
return lineElem.getEndOffset();
}
}
public int[] getLineOffsets(int line) throws BadLocationException {
int[] offsest = new int[2];
offsest[0] = getLineStartOffset(line);
offsest[1] = getLineEndOffset(line);
return offsest;
}
To calculate the line start and end positions, calculate the length of the text and remove it from the Document
, which might look more like...
int offset = ta.getCaretPosition();
int line = getLineByOffset(offset);
int[] lineOffsets = getLineOffsets(line);
int len = lineOffsets[1] - lineOffsets[0] - 1;
Document document = ta.getDocument();
String text = document.getText(lineOffsets[0], len);
document.remove(lineOffsets[0], len);