The setBackground
works fine. The problem is with the default BorderLayout
of the JFrame
. The only component you add is the Test
JPanel
which get expanded the size of the JFrame
, due the BorderLayout
, which ultimately covers up background color. If you take out the Test
JPanel
you will see the background color.
You can also see the affect, below, of setting the layout to GridBagLayout
to the frame and setting a preferredSize to the
JPanel`

import java.awt.Color;
import java.awt.Dimension;
import java.awt.GridBagLayout;
import javax.swing.*;
import javax.swing.border.TitledBorder;
public class Test extends JPanel {
public Test() {
setBorder(new TitledBorder("Panel size 300, 300"));
setBackground(Color.YELLOW);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(300, 300);
}
static void test() {
JFrame f = new JFrame("Test");
f.setLayout(new GridBagLayout());
f.getContentPane().setBackground(Color.blue);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.getContentPane().add(new Test());
f.setExtendedState(JFrame.MAXIMIZED_BOTH);
f.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
test();
}
});
}
}
The reason GridbagLayout
works is because it respects the preferredSize
of child components. As you can see from this answer, some Layout Managers respect the preferred sizes and some don't
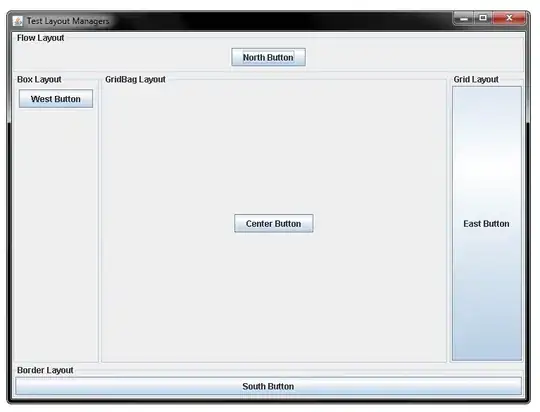