See Imshow subplots with the same colorbar and Set Colorbar Range in matplotlib.
If you use the same vmin
/vmax
and cmap
in all calls to imshow, the colors
should reflect the exact same intensities, regardless of the overall range in
the image.
Edit: added example after comments. It's possible that I've misinterpreted what the problem is but the code/image illustrate how the v limits can be set to match colors (top row) or what happens if each image is automatically normalised (bottom row).
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.cm as cm
offset = 5.5
x1 = np.random.rand(12,16) + offset
x2 = np.array(x1)
x2 = np.zeros((15, 20))
x2[2:2+x1.shape[0], 3:3+x1.shape[1]] = x1
vmin = x1.min()
vmax = x1.max()
cmap = cm.hot
plt.figure(1); plt.clf()
f, ax = plt.subplots(2,2, num=1)
im0 = ax[0,0].imshow(x1, interpolation='nearest', cmap=cmap, vmin=vmin, vmax=vmax)
f.colorbar(im0, ax=ax[0,0])
im1 = ax[0,1].imshow(x2, interpolation='nearest', cmap=cmap, vmin=vmin, vmax=vmax)
f.colorbar(im1, ax=ax[0,1])
ax[0,1].set_title('image with border, sharing v lims')
im2 = ax[1,0].imshow(x1, interpolation='nearest', cmap=cmap)
f.colorbar(im2, ax=ax[1,0])
im3 = ax[1,1].imshow(x2, interpolation='nearest', cmap=cmap)
f.colorbar(im3, ax=ax[1,1])
ax[1,1].set_title('image with border, own v lims')
f.show()
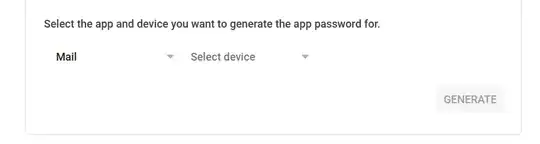
Note: the problem only manifests when the nonzero color range does not include zero. That is shown with the offset
variable above.