You can use two canvas elements instead:
(links just meant as examples)
An example of this could be including a simple basic draw function:
HTML:
<div id="cont">
<canvas id="canvasBottom" width=400 height=400></canvas>
<canvas id="canvasTop" width=400 height=400></canvas>
</div>
CSS:
#cont {
position:relative;
border:1px solid #777;
height:400px;
}
#cont > canvas {
position:absolute;
left:0;
top:0;
cursor:crosshair;
}
JavaScript:
Load and set the image to top layer:
var img = new Image,
canvas = document.getElementById('canvasBottom'),
canvasTop = document.getElementById('canvasTop'),
ctx = canvas.getContext('2d'),
ctxMouse = canvasTop.getContext('2d');
img.onload = setup;
img.src = 'http://i.imgur.com/HNiER0v.png';
ctx.lineWidth = 5;
ctx.strokeStyle = 'rgb(0, 100, 255)';
function setup() {
ctxMouse.drawImage(this, 0, 0);
}
Handle mouse:
var px, py, isDown = false;
canvasTop.onmousedown = function (e) {
var pos = getXY(e);
px = pos.x;
py = pos.y;
isDown = true;
}
canvasTop.onmouseup = function () {
isDown = false;
}
canvasTop.onmousemove = function (e) {
if (isDown) {
var pos = getXY(e);
ctx.beginPath();
ctx.moveTo(px, py);
ctx.lineTo(pos.x, pos.y);
ctx.stroke();
px = pos.x;
py = pos.y;
}
}
function getXY(e) {
var rect = canvasTop.getBoundingClientRect();
return {
x: e.clientX - rect.left,
y: e.clientY - rect.top
};
}
As you can see you can now draw behind the initial drawing:
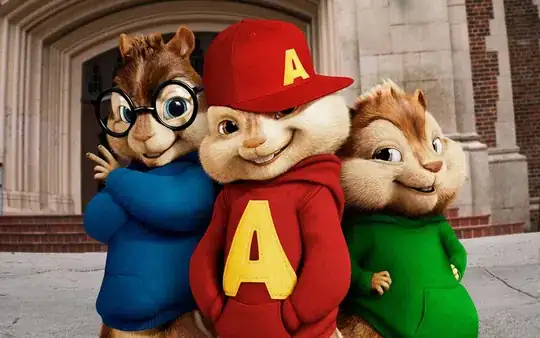
(Now, if that is not a master piece than I don't know what a master piece is..!)