After I found good matches, I draw the lines by using this code.
(...some code...)
//draw good matches
for( int i = 0; i < (int)good_matches.size(); i++ )
{
printf( "-- Good Match [%d] Keypoint 1: %d -- Keypoint 2: %d \n", i, good_matches[i].queryIdx, good_matches[i].trainIdx );
//query image is the first frame
Point2f point_old = keypoints_1[good_matches[i].queryIdx].pt;
//train image is the next frame that we want to find matched keypoints
Point2f point_new = keypoints_2[good_matches[i].trainIdx].pt;
//keypoint color for frame 1: RED
circle(img_1, point_old, 3, Scalar(0, 0, 255), 1);
circle(img_2, point_old, 3, Scalar(0, 0, 255), 1);
//keypoint color for frame 2: BLUE
circle(img_1, point_new, 3, Scalar(255, 0, 0), 1);
circle(img_2, point_new, 3, Scalar(255, 0, 0), 1);
//draw a line between keypoints
line(img_1, point_old, point_new, Scalar(0, 255, 0), 2, 8, 0);
line(img_2, point_old, point_new, Scalar(0, 255, 0), 2, 8, 0);
}
imwrite("directory/image1.jpg",img_1);
imwrite("directory/image2.jpg",img_2);
(...some code...)
I saved the results to the first (img_1) and next frame (img_2). As you see, I have different results, but the line shape is same. In video homography sample of OpenCV, keypoint tracking seems accurate. They follow this approach:detect keypoints-->compute keypoints-->warp keypoints--> match--> find homography-->draw matches. However, I apply detect keypoints-->compute keypoints-->match-->draw matches.
I am confused if I have to consider homography and perspective (or other things) to see the keypoint movements accurately.
my results for first frame (img_1)

and next frame (img_2)
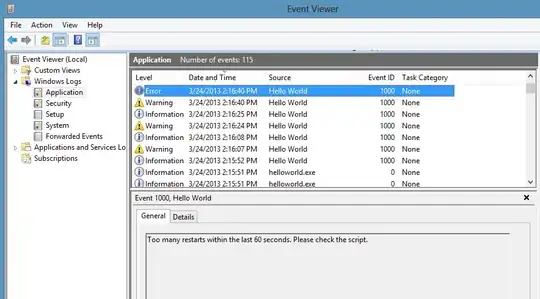