These are called lowercase numbers and can be turned on using UIFontDescriptor
.
First, you need to import CoreText for some constants:
#import <CoreText/SFNTLayoutTypes.h>
or
@import CoreText.SFNTLayoutTypes;
Then create font using font descriptor. Here I use Georgia family:
NSDictionary *lowercaseNumbers = @{
UIFontFeatureTypeIdentifierKey: @(kNumberCaseType),
UIFontFeatureSelectorIdentifierKey: @(kLowerCaseNumbersSelector),
};
UIFontDescriptor *descriptor = [[UIFontDescriptor alloc] initWithFontAttributes:
@{
UIFontDescriptorFamilyAttribute: @"Georgia",
UIFontDescriptorFeatureSettingsAttribute:@[ lowercaseNumbers ],
}];
UIFont *font = [UIFont fontWithDescriptor:descriptor size:15];
Result:
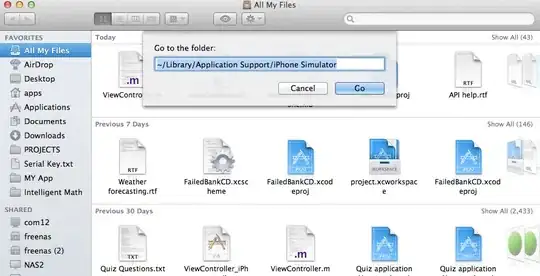
Edit: As @Random832 pointed out, Georgia has only lowercase numbers, so the result is irrelevant. However, @vikingosegundo confirmed this code works on supported fonts. Thanks.
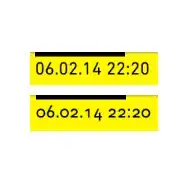
The top line was generated with
UIFont *font = [UIFont fontWithName:@"DIN Next LT Pro" size:12];
if (font)
label.font = font;
the second line with
NSDictionary *lowercaseNumbers = @{ UIFontFeatureTypeIdentifierKey:@(kNumberCaseType), UIFontFeatureSelectorIdentifierKey: @(kLowerCaseNumbersSelector)};
UIFontDescriptor *descriptor = [[UIFontDescriptor alloc] initWithFontAttributes:
@{UIFontDescriptorFamilyAttribute: @"DIN Next LT Pro",UIFontDescriptorFeatureSettingsAttribute:@[ lowercaseNumbers ]}];
UIFont *font = [UIFont fontWithDescriptor:descriptor size:12];
if (font)
label.font = font;