In a large project, keeping all views in a single storyboard may be daunting.
I prefer creating one storyboard per view; I modified the Container in Stuart's answer to look for a storyboard matching the view class, and fall back to the main storyboard if not found:
public class StoryBoardContainer : MvxTouchViewsContainer
{
protected override IMvxTouchView CreateViewOfType(Type viewType, MvxViewModelRequest request)
{
UIStoryboard storyboard;
try
{
storyboard = UIStoryboard.FromName(viewType.Name, null);
}
catch (Exception)
{
storyboard = UIStoryboard.FromName("StoryBoard", null);
}
return (IMvxTouchView) storyboard.InstantiateViewController(viewType.Name);
}
}
Caveat 1: To instantiate viewcontrollers this way, you must set the Storyboard ID in the editor:
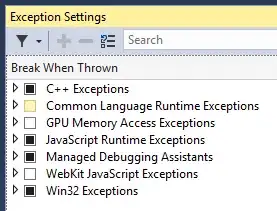
Caveat 2: Make sure your views inheriting MvxViewController
have the constructor public MyView(IntPtr handle) : base(handle)
, as this is used to instantiate the view controllers from the storyboard.