What you can do is override the prepareRenderer
method of the JTable
and set a tool tip for each cell. Then use some html for the tool tip as seen in this answer from AndrewThompson
I use this image this url https://i.stack.imgur.com/Bbnyg.jpg
from this site (this question), but you will probably want to use a resource from your system or class path and use toUri().toUrl()
to create the url. In any case, the html needs to consist of a URL in the <img src=
. You can switch them based on the row/column
value.
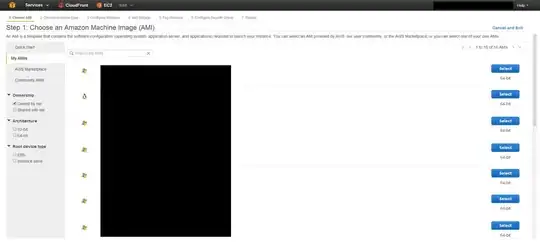
Here is the example.

import java.awt.Component;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.SwingUtilities;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableCellRenderer;
public class TestTableTooltip {
String html
= "<html><body>"
+ "<img src='"
+ "https://i.stack.imgur.com/Bbnyg.jpg"
+ "' width=160 height=120> ";
public TestTableTooltip() {
String[] cols = {"COL", "COL", "COL"};
String[][] data = {
{"Hello", "Hello", "Hello"},
{"Hello", "Hello", "Hello"},
{"Hello", "Hello", "Hello"}
};
DefaultTableModel model = new DefaultTableModel(data, cols);
JTable table = new JTable(model) {
public Component prepareRenderer(TableCellRenderer renderer, int row, int column) {
Component c = super.prepareRenderer(renderer, row, column);
if (c instanceof JComponent) {
JComponent jc = (JComponent) c;
jc.setToolTipText(html + "<br/>"
+ getValueAt(row, column).toString()
+ ": row, col (" + row + ", " + column + ")"
+ "</body></html>");
}
return c;
}
};
JFrame frame = new JFrame();
frame.add(new JScrollPane(table));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new TestTableTooltip();
}
});
}
}
UPDATE
You can get a url from a resource (in your class path) like this
URL url = getClass().getResource("/path/to/image.png");
final String html
= "<html><body>"
+ "<img src='"
+ url
+ "' width=150 height=150> ";
If it is a file from the file system, you can do this
URL url = new File("path/to/image.png").toURI().toURL();