Use collections.Counter
for creating the histogram data, and follow the example given here, i.e.:
from collections import Counter
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
# Read CSV file, get author names and counts.
df = pd.read_csv("books.csv", index_col="id")
counter = Counter(df['author'])
author_names = counter.keys()
author_counts = counter.values()
# Plot histogram using matplotlib bar().
indexes = np.arange(len(author_names))
width = 0.7
plt.bar(indexes, author_counts, width)
plt.xticks(indexes + width * 0.5, author_names)
plt.show()
With this test file:
$ cat books.csv
id,author,title,language
1,peter,t1,de
2,peter,t2,de
3,bob,t3,en
4,bob,t4,de
5,peter,t5,en
6,marianne,t6,jp
the code above creates the following graph:
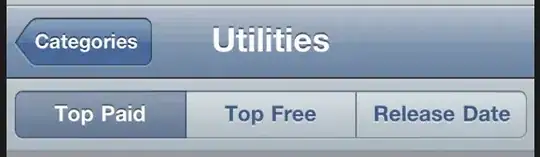
Edit:
You added a secondary condition, where the author column might contain multiple space-separated names. The following code handles this:
from itertools import chain
# Read CSV file, get
df = pd.read_csv("books2.csv", index_col="id")
authors_notflat = [a.split() for a in df['author']]
counter = Counter(chain.from_iterable(authors_notflat))
print counter
For this example:
$ cat books2.csv
id,author,title,language
1,peter harald,t1,de
2,peter harald,t2,de
3,bob,t3,en
4,bob,t4,de
5,peter,t5,en
6,marianne,t6,jp
it prints
$ python test.py
Counter({'peter': 3, 'bob': 2, 'harald': 2, 'marianne': 1})
Note that this code only works because strings are iterable.
This code is essentially free of pandas, except for the CSV-parsing part that led the DataFrame df
. If you need the default plot styling of pandas, then there also is a suggestion in the mentioned thread.