If I understand what you are asking correctly, I think you just need to have 2 height constraints for each of your subviews.
The first will be a height constraint with a constant of whatever you want the height to be when the parent view is shown, and set the priority to less than 1000 (I've been using 800, and it seems to work for what I want). This is the constraint that will be applied when the superview's height is NOT 0.
The second height constraint will have a constant
>= 0, and will have a priority
of 1000. This will be the constraint that will get applied when the superview height = 0.
EDIT: To get the height of a view for the priority 800 constraint, you can either:
Storyboard:
Set up the view with whatever content you want it to have. So for a label, set the number of lines, font size, etc., put in some text (it doesn't have to be exactly the text the user will see - your code will probably be changing myLabel.text
somewhere anyway. After the label is set up, click the "Pin" constraints button at the bottom right of the window, and the height should be auto-populated. Apply the constraint, and then edit the constraint after it has been created to lower the priority.
Code:
Setup the label's font, number of lines, and everything else like in the Storyboard approach, and add some dummy text again. Then, you can ask the label how much height it wants to display the dummy text you've given it by calling [myLabel sizeThatFits:CGSizeMake(CGFLOAT_MAX, CGFLOAT_MAX)].height
EDIT 2:
Okay, this is a little different than I had before. Let's try some screenshots and code snipets. These are just from a quick test project I put together, but you should be able to expand the idea to what you are working on.
In the storyboard, setup your horizontal constraints for your superview (green background) and for the location of the top of the superview.
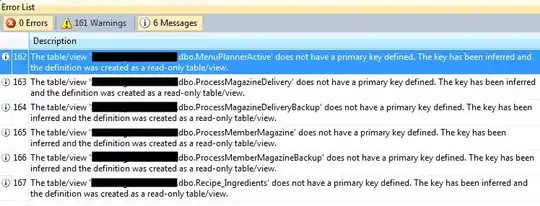
Then, add a height constraint for the expanded height. Set the Priority of this constraint to High
(750).
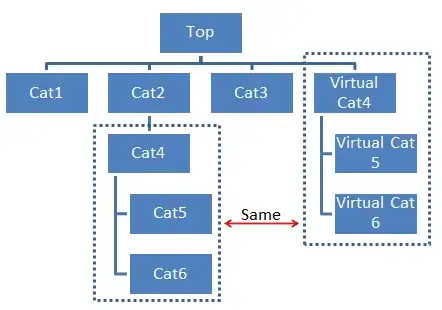
Then, add your subviews to the superview. Add constraints for the subviews as if everything was normal and nothing was going to be happening to the superview. The only difference is you want to set .hidden
to YES
by default (otherwise if the view starts collapsed the subviews will be visible and the spacing will be all funky). Note that for the multi-line label, I don't specify a height constraint - XCode will figure that out for itself based on the text I put in it.
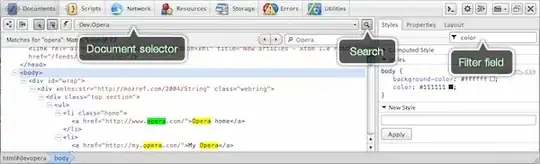
Lastly, add a second height constraint to the superview, with a constant
= 0 and a priority 1000.
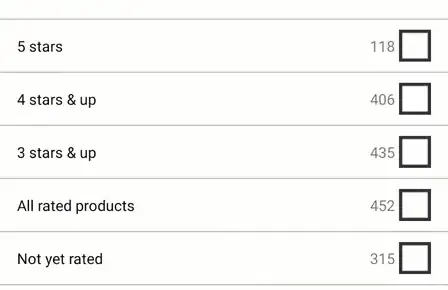
Now the little bit of Code:
In the .h file for the view controller, set up IBOutlet
s for the superview and for the height = 0 constraint of the view.
MyViewController.h
#import <UIKit/UIKit.h>
@interface MyViewController : UIViewController
{
IBOutlet NSLayoutConstraint *superHeighConstraint;
IBOutlet UIView *myCollapsibleView;
}
@end
For testing, I added a UISegmentedControl that will toggle if the superview is collapsed or not. Here is the code that responds to changes in selection:
MyViewController.m
...
- (IBAction)segmentUpdated:(UISegmentedControl *)sender
{
if ([sender selectedSegmentIndex] == 0)
{
[myCollapsibleView removeConstraint:superHeighConstraint];
for (UIView *subview in [myCollapsibleView subviews])
{
[subview setHidden:NO];
}
}
else if ([sender selectedSegmentIndex] == 1)
{
[myCollapsibleView addConstraint:superHeighConstraint];
for (UIView *subview in [myCollapsibleView subviews])
{
[subview setHidden:YES];
}
}
}
With this setup, you are never changing any of the constraints in the code - you are just choosing when to apply or not apply the height = 0 constraint of the superview. Looping through [myCollapsibleView subviews]
makes it easy to show/hide the subviews as needed, and it is done dynamically, so if you add or remove subviews later, you don't have to touch the code.
Simulator Pics:
Load:
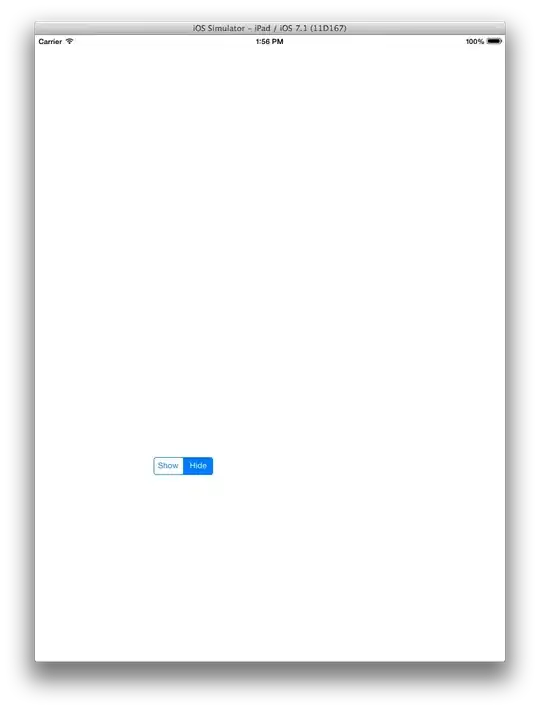
Toggle show:
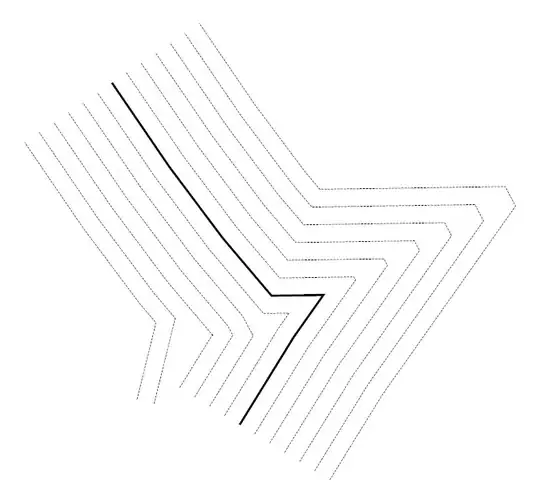
Toggle hide:
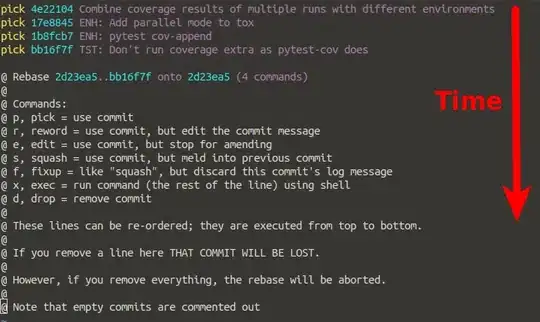