AFAIK the Minimum and Maximum buttons can be hidden as a group, but not individually.
Even back in the Windows Forms days, the MimimizeBox property worked this way.
this.MinimizeBox = false; // still visible, but disabled
this.MaximizeBox = false; // add this line and both buttons disappear
The System menu is a different story. It's possible to hide a menu item with this code.
CODE
public partial class MainWindow : Window {
private const int MF_BYPOSITION = 0x400;
[DllImport("User32")]
private static extern int RemoveMenu(IntPtr hMenu, int position, int flags);
[DllImport("User32")]
private static extern IntPtr GetSystemMenu(IntPtr hWnd, bool revert);
[DllImport("User32")]
private static extern int GetMenuItemCount(IntPtr hWnd);
private enum SystemMenu : int {
Restore,
Move,
Size,
Minimize,
Maximize
}
public MainWindow() {
InitializeComponent();
this.Loaded += MainWindow_Loaded;
}
private void MainWindow_Loaded(object sender, RoutedEventArgs e) {
WindowInteropHelper helper = new WindowInteropHelper(this);
IntPtr menuPtr = GetSystemMenu(helper.Handle, false);
int menuItemCount = GetMenuItemCount(menuPtr);
RemoveMenu(menuPtr, (int)SystemMenu.Minimize, MF_BYPOSITION);
}
}
Before Screenshot
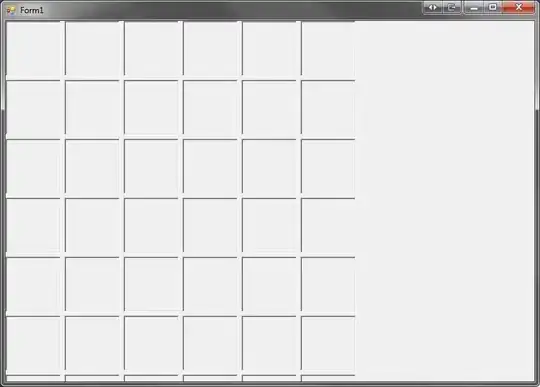
After Screenshot
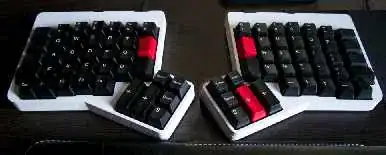
Using the
SetWindowLong(hwnd, GWL_STYLE, (currentStyle & ~WS_MINIMIZEBOX));
disables both the menu and the button.
This prevents the user from minimizing the tear off windows, but doesn't have the look you want.
I've seen replacement titles bars used to solve this issue, but its a lot of work to get right.