Another way to do this is by using regular expressions (Regex). See this link for more information on what the patterns mean.
Also, make sure you add ”Microsoft VBScript Regular Expressions 5.5″ as reference to your VBA module (see this link for how to do that).
I'm passing in a cell address, stripping out any letters, and then evaluating the function.
Function RegExpReplace(cell As Range, _
Optional ByVal IsGlobal As Boolean = True, _
Optional ByVal IsCaseSensitive As Boolean = True) As Variant
'Declaring the object
Dim objRegExp As Object
'Initializing an Instance
Set objRegExp = CreateObject("vbscript.regexp")
'Setting the Properties
objRegExp.Global = IsGlobal
objRegExp.pattern = "[a-zA-Z]"
objRegExp.IgnoreCase = Not IsCaseSensitive
'Execute the Replace Method
RegExpReplace = Evaluate(objRegExp.Replace(cell.Text, ""))
End Function
In Cell B1
enter this formula: =RegExpReplace(A1)
Results:
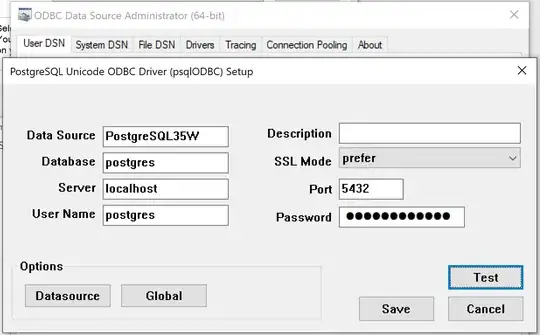